Lisp2D
Start of the interpreter
> ./lisp2d
Start without parameters enters into an interactive mode with the function read-eval-print.
> ./lisp2d filename [{ argi }]
By turns carries out all forms stored in a file filename. Supported UTF-8 encoding. Before executing of a file a variable arg links with the list of strings ("filename" "arg1" … "argn").
In all cases the received result of calculation comes back as a return code of the program if result was value of type char or integer, otherwise a return code - 0.
Interpreter uses a system multithreading, streams of the POSIX standard.
The quantity of working streams will be equal to quantity of processors. The quantity of calculations history records is 12. (For each of the parallel flow.)
The history of calculations at programming gives the big advantage. All knots of a course of the program are visible and the site in which there was an unexpected operation is visible. Speed of a program debugging increases 10 times.
(nil setdebug),(nil setdebug false),(nil setdebug 0) - cancels record in history of calculations. Speed of the program increases.
(nil setdebug number) - changes the size of history of calculations.
(nil getdebug) - returns the size of history of calculations.
Syntax
(obj … obj) - list
#(obj … obj) vector (array)
#[byte … byte] – vector (array) of bytes
-
"ab…z" - string
-
The string having special characters is created by means of a sign \
"the \"abc\" is string" - it is one string
"\\" - string from one character \
;abc…z - the comment up to the end of a line
'obj - prohibition of calculations.
`obj - back quote (partial prohibition of calculations).
,obj - calculation inside `.
,@obj - calculation with an insertion inside `.
(obj . obj) - dot pair
-
#\c - letter (character) c
-
#\null - end of string
#\space - space
#\tab - tabulator
#\vtab - vertical tabulator
#\newline
#\backspace
#\return - carriage return
#\feed - form feed
#\bell
#\escape
Keywords and variable environments
A keyword can be put in the list of arguments before the variable name.
-
&rest - Connects a variable with the list of the staying arguments
-
(nil defmethod m (a &rest b) (cout writeln a b))
(nil m 1 2 3 4) →
1
(2 3 4)
-
&whole - connects a variable with an original call where are not calculated neither object nor arguments.
-
>(nil defmethod t (&whole w) (cout writeln "w=" w))
(lambda (&whole w) (cout writeln "w=" w))
>((10 + 10) t)
w=((10 + 10) t)
-
&const - protection against changes in the value of the variable. In the area of its visibility change due to another atom leads to the exclusion. Protection of the domestic content of the atom (numbers, strings, etc.) is done by using the (nil const obj).
-
(nil defmethod t (&const c) ('c set 0) …)
(nil t 1) → exception
nil nothing or empty list
true- The logic truth
false - The logic false
Global variables:
package - The variable, contacts a loaded file
arg - the variable, link to the list of strings, transmitted at interpreter starting.
pi=3.141592653589… π
e=2.718281828459…
cin - standard stream of input
cout - standard stream of an output
cerr - standard stream for error messages.
xk-enter, xk-home, xk-end, xk-left, xk-right, xk-up, xk-down, xk-page-up, xk-page-down, xk-insert, xk-delete, xk-caps-lock, xk-shift-l, xk-shift-r, xk-control-l, xk-control-r, xk-alt-l, xk-alt-r, xk-f1, xk-f2, xk-f3, xk-f4, xk-f5, xk-f6, xk-f7, xk-f8, xk-f9, xk-f10, xk-f11, xk-f12, xk-backspace, xk-undo, xk-num-lock, xk-kp-multiply, xk-space, xk-numbersign, xk-question - codes of the pressed keys in window application
lambda or λ - as the first character in the list shows that the entire list of this definition of an anonymous function. Practically, this may simply be a list of words. To run function or not, decides the algorithm of program.
In objects:
this - value of itself (object)
socket-max-connections - the number can be set to 128
(object man [method]) - returns documentation string
Types
-
(nil type-of obj) - returns a symbol designating type of result of calculation obj, for objects returns a classname
-
nil - nothing (the empty list)
boolean - logical.
cons - List cell
btree - a balanced binary tree
btree-node - node of a balanced binary tree
vector - vector (array)
byte-vector - vector (array) of bytes
string - string of characters
number - Number (only one type of numbers)
symbol - Symbol
char - character
stream - Stream of an input / output
stat - File state structure
socket - communication between computers or processes.
window - really window
time - the date and time.
dir - directory processor.
bif - built-in kernel function.
lock
signal
environment
quote - '
backquote - `
unquote - ,
unquote-splicing - ,@
At definition of types it is better to use logic functions:
-
(nil atom obj) - obj is not a list cell
-
(nil atom nil) → true
(nil atom false) → true
(nil atom cons) → false
(nil atom obj) → obj
-
(nil = {obj}) - all obj are nil
-
(nil = false) → false
(nil = nil) → true
(nil = true) → false
-
(nil <> {obj}) - none obj is not equal nil
-
(nil <> false) → true
(nil <> nil) → false
(nil <> true) → true
-
(false = {obj}) - equality false
-
(false = false) → true
(false = nil) → false
(false = true) → false
-
(false <> {obj}) - none obj is not equal false
-
(false <> false) → false
(false <> nil) → true
(false <> true) → true
-
(true = {obj}) - equality true
-
(true = false) → false
(true = nil) → false
(true = true) → true
(true = 12) → false
-
(true <> {obj}) - none obj is not equal true
-
(true <> false) → true
(true <> nil) → true
(true <> true) → false
-
(nil booleanp obj) - returns true if obj is true or false.
-
(nil booleanp false) → true
(nil booleanp 1) → false
-
(nil stringp obj) - obj is string
-
(nil stringp "ab") → "ab"
(nil stringp 1) → false
-
(nil charp x) - character
-
(nil charp #\t) → #\t
(nil charp true) → false
-
(nil listp obj) - of conformity to a list cell or nil
-
(nil listp nil) → true
(nil listp 1) → false
(nil listp '(1 . 2)) → (1 . 2)
-
(nil consp obj) - of conformity to a list cell
-
(nil consp 1) → false
(nil consp '(1)) → (1)
(nil btreep obj) - of conformity to a balanced binary tree. Returns tree or false.
(nil btree-nodep obj) - of conformity to a node of balanced binary tree. Returns node or false.
-
(nil vectorp obj) - of conformity to a vector
-
(nil vectorp #(1 2)) → #(1 2)
(nil vectorp 1) → false
-
(nil byte-vectorp obj) - of conformity to a byte-vector
-
(nil byte-vectorp #[1 2]) → #[1 2]
(nil byte-vectorp 1) → false
(nil symbolp obj) - of conformity to a symbol
(nil numberp obj) - number
-
(nil streamp obj) - stream
-
(nil streamp notstream)→false
(nil streamp stream)→stream
(nil statp [x]) - if x is struct stat, returns x, else false.
(nil socketp obj) – communication between computers or processes.
(nil objectp obj) - object of a class
-
(nil windowp x) - conformity to a window
-
(nil windowp win) → win
(nil windowp 1) → false
(nil timep obj)
(nil dirp obj)
(nil bifp obj)
(nil lockp obj)
(nil signalp obj)
(nil envp obj)
(nil quotep obj)
(quote setf quote)
(quote swap quote)
(nil backquotep obj)
(backquote setf backquote)
(backquote swap backquote)
(nil unquotep obj)
(unquote setf unquote)
(unquote swap unquote)
(nil unquote-splicingp obj)
(unquote-splicing setf unquote-splicing)
(unquote-splicing swap unquote-splicing)
Symbols
Symbols it is auxiliary object for programming, Pure Lisp have no symbols. They can have values: usual and functional, at them is present auxiliary properties.
-
(symb <> [s1 … sn]) - any symbol si is not equal symb
-
(nil not (symb <> [s1 … sn])) - at last one of the symbols si is equal to main object symb
(symb = [symb…symb]), (symb < [symb…symb]), (symb <= [symb…symb]), (symb > [symb…symb]), (symb >= [symb…symb]) - comparisons (alphabetically).
(symb min symb … symb), (symb max symb … symb) - minimal/maximal (alphabetically).
-
(symb + {symb|string|char}) - new symbol
-
(('abc + '- 'def) set 1)=('abc-def set 1)
-
(symb - symb … symb) new symbol
-
(('abcdef - 'cd) set 1)=('abef set 1)
(string|char symbol) - returns a symbol with a name string|char.
-
(symbol put prop val) - establishes property prop a symbol symbol with value val, returns val
-
Property of a symbol is only in the current space of names.
('work1 namespace
(symbol put prop propv))
('work2 namespace
(symbol get prop)) → NIL
-
(symb get prop) - property of a symbol symb with a name prop. At absence of the given property comes back NIL
-
At ocurrence of several spaces of names the first got property comes back.
('std namespace
(symb put prop propv)
…
('another namespace
(symb get prop) → propv
…))
(symb remprop prop) - deletes property of a symbol symb with a name prop. If deleted property is not present - comes back false differently true. Property deletes only in current space of names.
(symb properties) - returns all properties of a symbol as #((symb. value) …), pairs in vector are sorted on symb
(symbol boundp [env]) - whether symbol has a value. Returns true or false.
(nil|symbol gensym) - Function-generator of the different symbols beginning on symbol if the initial symbol is not specified begins on t. It is guaranteed, that the returned symbol is new.
Constants
The variable can be set to have a constant property. Designed for clarity algorithm which variables do not need to change. When you try to change the value of the variable will be an exception. Being a constant condition is temporary, you can cancel the constancy, check property variable.
-
(symbol const) - sets the variable flag constants. It returns the symbol in the success of an exception in the absence of the variable value.
-
('x set (nil localtime))
('x const)
('x set 0) → exception
('y const) → exception
-
(symbol unconst) - cancels the variable flag constants.
It returns the symbol in the success of an exception in the absence of the variable value.
-
('pi unconst)
('pi set "Me")
('x unconst) → exception
-
(symbol constp) - check the variable flag constants.Returns true, false or exclusion in the absence of the variable value.
-
('x set 0)
('x constp) → false
('pi constp) → true
('y constp) → exception
To the atom it is possible to put a flag constants (and not link to it). It is used when using a change of atomic object. (functions: += , setf , swap , move , setfirst , setrest , resize , setelt , etc.)
When you try to change a constant atom gives an exception.
(nil const obj) - puts to the atom flag constant.
(nil unconst obj) - cancels property of constant. Leads to an error when using to the argument: nil, false, true or any symbol.
(nil constp obj) -
returns a true if obj is a constant or else false.
Setting the value
(nil let (([&const] var1 [{body1}])|var1…([&const] varn [{bodyn}])|varn) [{main-body}]) - creates in parallel local links of variables vari with values (nil progn [{bodyi}]) and by turns calculates the form main-body. Variables without value [{bodyi}] are initialized with value nil. If before the variable name is the symbol &const further change in values will lead to exclusion.
-
(symbol set val [env]) - changes the value of the variable. If the variable has not, it is created in the current environment. Returns value val.
-
(('(x y) first) set 1)≈(nil setq x 1)
-
(var letset val) - giving of values of a variable var in the current environment (does not touch value of a variable above). Name of a variable var is calculated. Result will be val
-
It is applied at lateness of definition of variables of the form let:
('x set 1)
(nil let ()
…
('x letset -2)
…)
x → 1
-
(nil|environment setq [{symb val}]) - changes the value of variables. If some variable has not, it is created in the current environment. The symbols symb are not calculated. Arguments val are computed in parallel. Returns nil.
-
(nil setq x (10 - 1) y (2 / 3))
x → 9
y → 2/3
(nil environment) - current environment.
(nil environment-external) - external environment.
Forms of management
(nil progn b1 … bn) - by turns calculation b1 … bn , returns result of calculation bn after calculation of all forms b1…bn.
-
(nil parallel task … task) - parallel calculation of task's. After all tasks are completed, nil is returned.
(nil fork {body}) - creates new task (nil progn {body}), does not expect their result and returns nil. The main task can not catch exceptions of subtask.
-
(nil if test then else [uncert]) - calculates the test, if the result is affirmative object (not false and not nil), then further action will be the calculation of the body then. If the result of evaluating test will false, will be performed else. The remaining variant is the result of nil, in the presence of a the task uncert will execute it, or otherwise else.
-
(nil if) →nil
(nil if test)=(nil progn test nil)
(nil if true 1 2 3) → 1
(nil if false 1 2 3) → 2
(nil if nil 1 2 3) → 3
(nil if nil 1 2) → 2
-
(nil when test {work})=(nil if test (nil progn {work})) - if result of test calculation is positive, calculates by turns tasks work.
-
(nil cond (test1 [{form1}])|atom … (testn [{formn}])|atom) - by turns calculated testi while not found not false and not nil value. Returns result of last calculation of the corresponding form formi. If formi it is not specified, the result of calculation testi comes back. Providing arguments: atoms - are ignored.
-
(nil cond (false 1) ignore) → nil
(nil cond (false 1) (nil (cout write …))) → nil
(nil cond (false 1) (ok oking)) → evaling oking
(nil cond (false 1) (ok)) → ok
(nil cond (false 1) (notok oking) (true default)) → evaling default
'obj - calculation of obj is blocked.
(nil|environment eval obj) - double calculation. First obj it is calculated as argument of function eval , then returns the result of calculation by the interpreter of the received value in a corresponding environment.
(nil|environment apply obj fn arglist) - application of function fn to arguments arglist. Value of a variable fn can be a name or lambda-list. Arguments and object calculates only once.
(nil|environment funcall obj fn [{arg}]) - application of function fn to arguments arg. Value of a variable fn can be a name or lambda-list. Arguments and object calculates only once.
-
`obj - partial prohibition of calculations of argument obj . When inside the form obj there is a ,x - that subform x is calculated and substituted in the form obj . Also there is a ,@x - it means that value x as the list is substituted by elements in the form obj.
-
('l set '(1 2 . 3))
`(0 ,l ,@l)→(0 (1 2 . 3) 1 2)
-
,obj - insertion inside the form `.
-
,@obj - insertion with distribution inside the form `.
-
`(a ,@'(1 + 1) b c)→(a 1 + 1 b c)
(string load [arg1 … argn]) - reads out a file with a name string, calculates and returns corresponding result. Before calculation the variable arg links with the list of arguments (arg1 … argn). After calculation the previous value arg is restored.
(string save obj) - writes down object obj in a format of writing in a file with a name string.
-
(nil do-while {body} test) - cyclic calculation of the forms body, if its last result will be nil or false calculations stop and result will be this value.
-
To stop the cycle inside the body more comfortable using the exceptions.
(nil try (nil do-while … (nil throw 'break) … ) break last-task)
-
(nil while test {body}) - by turns conditional cycle. It is checked test, if test=false or nil then result will be this value. Then forms are by turns carried out body, then a step to check test.
-
To stop the cycle inside the body more comfortable using the exceptions.
(nil try (nil while … (nil throw 'break) … ) break last-task)
-
(nil try task-body {exception-name exception-body}) - running task-body. While task running an exception was occurred, the exception-body is running. The exception-name is not calculated. Name verifying makes by equality eq. If is not supplied the name of current exception, will not executed additional tasks and an exception keeped. The last name may be nil, then any exception will be work up.
-
(nil try (nil setq result (nil eval task)) nil (nil setq error true)) - when by interpreting task was an error, the flag error is set.
-
(nil throw [{exception}]) - argument calculating, exception setting.
-
(nil throw) → nil
(nil throw x) → x value
(nil throw x y) → list of x and y values
-
(nil exception) - the current exception is returned.
-
(nil try (nil eval task) nil (cerr writeln "exception: " (nil exception)))
-
(nil exception-history) - returns the history of messages in the exception time. Out of block try returns nil. The size of the message history for each parallel thread is limited. (q.v. getdebug and setdebug)
-
(nil try (nil eval task)
nil (nil progn
(cerr writeln "exception-history: ")
((nil exception-history) for* history-i (cerr print history-i))
)
)
-
(nil log [{obj}]) - the obj arguments are evaluated. An element is added to the calculation history as a list of the results of the obj arguments. Nil is returned.
To get the current message list, you can use the log-list method.
-
-
(nil log-list) - returns a list of saved messages (created with log). Not intended to handle exceptions. (q.v. exception-history) The size of the message history for each parallel thread is limited. (q.v. getdebug and setdebug)
-
If there are exceptions, messages of parallel threads are not visible. Neither old messages nor new ones (after an exception in this thread). Only the parent ones are visible. When you manually call log-list, logistic information will not be removed from many other streams. Each successive thread has a message limit. Only the last will be visible.
This assembly of information is intended only for a temporary search for a program error. The global assembly of all messages from all threads will lead to a slowdown in their work and a huge amount of information.
Logic
Three-valued logic is defined using objects nil, false, true. Symbol true - defines truth, false - falsehood, nil - indeterminacy. Any other object is presented as truth.
-
(nil not obj)=(nil ¬ obj) - simply not. ¬ = ((172 char) symbol)
-
x | not x |
false | true |
nil | nil |
true | false |
-
(nil not nil) → nil
(nil not false) → true
(nil not true) → false
(nil not 1) → false
-
(nil or a b … z)=(nil ∨ a b … z) - selects the maximum value in the order of: false → nil → true. ∨ = ((8744 char) symbol)
-
x or y | false | nil | true |
false | false | nil | true |
nil | nil | nil | true |
true | true | true | true |
-
(nil or) → false
(nil or nil) → nil
(nil or true) → true
(nil or false) → false
(nil or 1) → 1
(nil or false nil) → nil
(nil or false nil 123) → 123
- (nil nor a b … z)=(nil ⊽ a b … z)=(nil not (nil or a b … z))=(nil and {(nil not …)}) - if in the arguments will fall affirmative returns false. If all arguments is false returns true. In the remaining event with
arguments nil returns nil. ⊽ = ((8893 char) symbol)
-
x nor y | false | nil | true |
false | true | nil | false |
nil | nil | nil | false |
true | false | false | false |
-
(nil and [a [b … [z]]])=(nil ∧ [a [b … [z]]]) - selects the minimal value in the order of: true → nil → false. ∧ = ((8743 char) symbol)
-
x and y | false | nil | true |
false | false | false | false |
nil | false | nil | nil |
true | false | nil | true |
-
(nil and) → true
(nil and nil) → nil
(nil and false) → false
(nil and 123) → true
(nil and 123 nil) → nil
(nil and 123 nil false) → false
-
(nil nand [a [b … [z]]])=(nil ⊼ [a [b … [z]]])=(nil not (nil and [a [b … [z]]]))=(nil or {(nil not …)}) - if in the arguments will fall false returns true. If all arguments affirmative returns false. In the remaining event with arguments nil returns nil. ⊼ = ((8892 char) symbol)
-
x nand y | false | nil | true |
false | true | true | true |
nil | true | nil | nil |
true | true | nil | false |
-
(nil xor a b … z)=(nil ⊻ a b … z) - returns a single affirmative value. If you came across more than one returned false. If there are arguments nil - the result is nil. ⊻ = ((8891 char) symbol)
-
x xor y | false | nil | true |
false | false | nil | true |
nil | nil | nil | nil |
true | true | nil | false |
-
(nil xor) → false
(nil xor nil) → nil
(nil xor false) → false
(nil xor 123) → 123
(nil xor false false) → false
(nil xor false nil) → nil
(nil xor false 123) → 123
(nil xor nil nil) → nil
(nil xor 123 234) → false
(nil xor nil 123) → nil
(nil xor false 1 false false) → 1
(nil xor false 1 false nil) → nil
(nil xor false 1 false 2) → false
-
(nil imply x y)=(nil -> x y) - implication.
-
x → y | false | nil | true |
false | true | true | true |
nil | nil | nil | true |
true | false | nil | true |
-
(nil eq a b) - physical equality of two objects (equality of pointers)
-
(nil eq) → true
(nil eq a) → (nil = a)
(nil eq nil nil) → true
(nil eq false false) → true
(nil eq 1 1) → false
(nil eq 'sym 'sym) → sym
Numbers
12 - integer.
12.3 - with fraction part.
2/3 - fraction.
1/2/3 - one integer plus two-thirds.
1/2/3e20=(1+2/(3*1020))=(1+1/(15*1019)) - with the exponential.
1+2/3i, 1/3i, -7i+1e100 - complex.
-
(a + b c … z) - addition a+b+c+…+z.
-
(a +) → a
(a + 1 2) → a+3
(2/3 + 1) → 1/2/3
(1+2i + 1+1i) → 2+3i
-
(a - b c … z) - subtraction a-b-c-…-z.
-
(a -) → -a
(a - 1 2) → a-3
(2/3 - 1) → -1/3
(1+2i - 1+1i) → 1i
-
(a * b … z) - multiplication.
-
(a *) → a
(a * 2 3) → a*6
(1+2i * 2i) → -4+2i
-
(a / b … z) - division on all other numbers.
-
(a /) → 1/a
(a / 2 3) → a/6
(1 / 2/3) → 1.5
(1 / 1+2i) → 0.2-0.4i
-
(a = b … z) - equality a=b=…=z.
-
(2 = 2) → 2
(2 = 3) → false
-
(n <> x1 … xk) - any number xi is not equal n.
-
(nil not (n <> x y z)) - number is equal to one of the arguments.
(a < b … z) - numbers grow a<b<…<z. Returns object (number) or false or nil.
(a > b … z) - numbers decrease a>b>…>z. Returns object (number) or false or nil.
(a <= b … z) - less or equal (do not decrease) a≤b≤…≤z. Returns object (number) or false or nil.
(a >= b … z) - bigger or equal (do not grow) a≥b≥…≥z. Returns object (number) or false or nil.
(x nanp) - predicate of 0/0.
(x infp) - predicate of ±∞.
(x finitep) - predicate of finite number, not ±∞ and not 0/0.
-
(number integerp) - integer number.
-
(1.5 integerp) → false
(2 integerp) → 2
(1/0 integerp) → nil
-
(number evenp) - predicate, on evenness.
-
(3 evenp) → false
(-4 evenp) → -4
(3.5 evenp) → nil
-
(number oddp) - predicate, on oddness.
-
(4 oddp) → false
(-5 oddp) → -5
(3.5 oddp) → nil
(numb min numb … numb), (numb max numb … numb) - the minimal/maximal number.
-
(a abs) - absolute value.
-
(2 abs) → 2
(-3 abs) → 3
(3+4i abs) → 5
-
(x ceil [d]) - the minimal integer, not smaller x. In the complex number, rounding is in the real part and in the imaginary part.
-
(-2.5 ceil) → -2
(2.5 ceil) → 3
(7 ceil 2)=((7 / 2) ceil) → 4
(2 ceil) → 2
(4.5+3.5i ceil) → 5+4i
(4.5+3.5i ceil 2-1i) → 2+3i
-
(x floor [y]) - rounding off x in the smaller side up to an integer. If given y then returns the integer part from division x on y. In the complex number, rounding is in the real part and in the imaginary part.
-
(-2.5 floor) → -3
(13 floor 4) → 3
(-13 floor 4) → -4
(4.5+3.5i floor) → 4+3i
(4.5+3.5i floor 2-1i) → 1+2i
-
(x trunc [y]) - rounding x too the nearest integer number in the direction of zero. If given y then returns the function result from division x on y. In the complex number, rounding is in the real part and in the imaginary part.
-
(2.5 trunc) → 2
(-2.5 trunc) → -2
(13 trunc 4) → 3
(-13 trunc 4) → -3
(4.5+3.5i trunc) → 4+3i
(-4.5-3.5i trunc 2-1i) → -1-2i
-
(x % [y])=(x mod [y]) - the rest of division x on y.
-
(13 % 4) → 1
(-13 % 4) → 3
(13 % -4) → -3
(-13 % -4) → -1
(13.4 % 1) → 0.4
(-13.4 % 1) → 0.6
(2.3 %)=(2.3 % 1) → 0.3
(2.5+3.5i %) → 0.5+0.5i
(2.5+3.5i % 1i) → -0.5+0.5i
-
(x gcd [{xi}]) - the greatest common divider of integers, returns a positive integer.
-
(3 gcd)=3
(-10 gcd) =10
(12 gcd -8) =4
(32 gcd 16 3) =1
-
(x lcm [{xi}]) - the minimum common multiple of integers, returns a positive integer.
-
(3 lcm)=3
(-10 lcm) =10
(12 lcm -8) =24
(32 lcm 16 3) =96
-
(x sin [eps]) – sine with the accuracy eps>0.
[result-eps…result+eps]
-
By default, the precision is 2^-64. Log10(2^64) = 19 digits.
(1 sin) → 17152820301/20384327696
(1 sin 0.1) → 5/6
-
(x cos [eps]) – cosine with the accuracy eps>0.
[result-eps…result+eps]
-
By default, the precision is 2^-64. Log10(2^64) = 19 digits.
(1 cos) → 3559790192/6588515639
(1 cos 0.1) → 0.5
(x tan) - a tangent.
(x cot) - cotangent.
(x arcsin)=sin-1x
(x arccos)=cos-1x
(x arctan)=tg-1x
(x arctan y)=tg-1(x/y) ,y>0 ,x>0
(x sinh)=(ex-e-x)/2
(x cosh)=(ex+e-x)/2
(x tanh)=sinh(x)/cosh(x)
(x coth)=cosh(x)/sinh(x)
(x arsinh)=sinh-1x
(x arcosh)=cosh-1x
(x artanh)=tanh-1x
-
(x sqrt [eps])=(x √ [eps]) - square root √x with the relative accuracy eps>0.
[result*(1-eps)…result*(1+eps)]
-
By default, the precision is 2^-64. Log10(2^64) = 19 digits.
(x sqrt 1) ≈ Floor[sqrt(x)]
(2 sqrt 0.1) → 1.4
(2 sqrt) → 1/3166815962/7645370045
High precision for calculations takes more time.
(2 sqrt 1e-38) → 1/4866752642924153522/11749380235262596085
There are two results, returned positive.
(9 sqrt) → 3
Another: -3
(8i sqrt) → 2+2i
Another: -2-2i
-
(x cbrt [eps])=(x ∛ [eps]) - cube root ∛x with the relative accuracy eps>0.
[result*(1-eps)…result*(1+eps)]
-
(2 cbrt) → 1/914705237/3519165675
By default, the precision is 2^-64. Log10(2^64) = 19 digits.
(2 cbrt 0.1) → 1.25
There are three results. If the number is not imaginary, returns are not imaginary result.
(8 cbrt) → 2
If the number is purely imaginary (real=0), returns a purely imaginary result.
(8i cbrt) → -2i
To get all three results must be turned at an angle ⅔π of a complex number.
(nil let ((x (2-2i cbrt)))
(cout writeln x)
(cout writeln ((x abs) polar ((x arg) + (pi * 2/3))))
(cout writeln ((x abs) polar ((x arg) - (pi * 2/3))))) →
-1-1i
1.36602540378-0.366025403784i
-0.366025403784+1.36602540378i
(x exp) - exponent ex
(b ^ n) - a degree bn
(x log [a])=logax - the logarithm on the basis a (by default natural logarithm).
-
(x ln [eps]) - natural logarithm with the accuracy eps.[result-eps…result+eps] By default, the precision is 2^-64. Log10(2^64) = 19 digits.
-
(2 ln) → 6350113169/9161276778
(2 ln 0.1) → 5/7
-
(n string [digc]) - returns the string, which precisely represents number. Can be in a fractional form. The maximum quantity of digits digc is if necessary established.
-
(4/6 string) → "2/3"
(4/6 string 2) → "6.7e-1"
(4/6 string 0) → "6.66666666667e-1" ; 12 digits
(x !)=1*2*…*x - a factorial.
-
(nil random) - returns random floating number, in an interval [0…1).
-
(nil random) → 0.102355897908
-
(numb random) - returns random floating number, smaller on absolute value, than numb.
-
(pi random) → 2.0939049352352
(-3 random) → -1.194246236960
-
(numb randomcomplex) - returns a random complex number, smaller in absolute value than abs|numb|.
The distribution is uniform.
-
(10 randomcomplex) → -5.74660815753-7.37486568128i
(10i randomcomplex) → 0.108014340751-4.54071316933i
-
(average random-normal deviation) - returns random number, with normal distribution.
-
(0 random-normal 1) → -0.1411..
(0 random-normal 1i) → -0.2241..i
(1+1i random-normal 1+1i) → 0.8483..+0.8483..i
-
(number for symbol {body})=(number ∀ symbol {body}) - in parallel local variables symbol (for each process the personal variable symbol) numbers are given 0…number-1, at each value forms are calculated by turns body. ∀=((8704 char) symbol)
-
(3 for i (cout write " i+1=" (i + 1)) (i + 1)) →
i+1=2 i+1=1 i+1=3
→ nil
To stop the cycle inside the body more comfortable using the exceptions.
(nil try (number for … (nil throw 'break) … ) break last-task)
-
(number for* symbol {body}) - by turns local variable symbol gives numbers 0…number-1, forms body are calculated by turns. Last result of the form body comes back.
-
(3 for* i (cout writeln "i=" i "..")) →
i=0..
i=1..
i=2..
To stop the cycle inside the body more comfortable using the exceptions.
(nil try (number for* … (nil throw 'break) … ) break last-task)
-
(number setf number)=(number number number) - physically changes number with new value. Returns the modified object. Using the of this operation prone to error programming.
-
('x set 7) → 7
('y set (nil list x x x)) → (7 7 7)
(x setf (x - 1)) → 6
y → (6 6 6)
For avoidance of damage of the working program it is necessary to use function of copying:
(nil let ((x 0)) … → (nil let ((x (0 copy))) …
('x set 0) → (x setf 0)
(nil setq x 0) → (x setf 0)
-
(number swap number) - physically changes values of numbers. The change is very fast. Using the of this operation prone to error programming.
-
('x set 3) → 3
('y set 4) → 4
('z set (nil list x y)) → (3 4)
(x swap y) → 4
x → 4
y → 3
z → (4 3)
-
(number copy) - returns new number.
-
('number newobject)≈(0 copy)
('number newobject number)=(number copy)
('number newobject number number) → C(number number)
-
(number += [{x}])≈(number setf (number + [{x}]))
-
(number -= [{x}])≈(number setf (number - [{x}]))
-
(number *= [{number}])≈(number setf (number * [{number}]))
-
(number /= [{number}])≈(number setf (number / [{number}]))
-
(number degrees)=number*180/π
-
(number radians)=number/180*π
-
(n combin x)=C(x,n)=n!/x!/(n-x)!
-
(n combina x)=n!/(n-x)!
-
(x multinomial y … z)=(x+y+…+z)!/x!/y!…/z!
-
(number round [diff]) - returns roundoff: [number-diff … number+diff]
-
>(pi round 1e-3)
3/15/106
>(e round 1e-3)
2.71875 ; = 2/23/32
(x round 1) = [x]
(x round)=(x round 0.5) = [x+0.5]
-
(number num) - positive integer numerator of number.
-
(number denom) - positive integer denominator of number.
-
(number real) - real part of number. x+yi → x
-
(number imag) - the imaginary part of number. x+yi → y
-
(number conj) - conjugate of number. x+yi → x-yi
-
(abs polar arg) - number in trigonometric form. abs*(cos(arg)+i*sin(arg))
-
(number arg) - argument arg(number) number -π…π
-
(number erf) - error function of normal distribution (Gauss):
erf(x) = 2/√π∫0x(ⅇ-t2)ⅆt returns number [-1..1]
-
(number inverf) - inverted error function of normal distribution from [-1..1] to [-∞..∞] : 0.999979 → 3
List
-
(list first) - returns a first element of the list.
-
('(1 2 3) first)→1
(nil first)→nil
-
(list rest) - returns a tail part of the list.
-
('(1 2 3) rest) → (2 3)
(nil rest)→nil
(list second)=((list rest) first)
(list third)=(((list rest) rest) first)
-
(list size) - returns length of the list (count of list cells).
-
(nil size) → 0
(nil cons first rest) - creates a list cell with respective links.
('cons newobject first rest)=(nil cons first rest)
('cons newobject another-cons)=(another-cons copy)
('cons newobject)=(nil cons nil nil)
-
(list elt [{integer}]) - returns an element of the list listinteger,…. Elements of the list are numbered: 0 - first(list) 1 - second(list) … (size-1) – first(last(list)).
-
('((1 2) (3 4)) elt) → ((1 2) (3 4))
('((1 2) (3 4)) elt 0) → (1 2)
('((1 2) (3 4)) elt 0 1) → 2
('((1 2) (3 4)) elt 0 1 2) → nil
(nil list arg1 … argn) - returns the list of arguments (arg1 … argn), arguments are calculated in parallel
-
(nil list* arg1 … argn) - returns the list of arguments (arg1 … argn-1 . argn), last argument incorporates as tail, arguments are calculated in parallel
-
(nil list* 'a 'b '(c d e)) → (a b c d e)
-
(list last) - returns last list cell
-
('(1 2 3) last) → (3)
('(1 2 . 3) last) → (2 . 3)
(nil last) → nil
-
(list + [{list}]) - returns concatenation of lists list. All lists do not destroyed.
-
('(1 2 3) + '(4 5 6)) → (1 2 3 4 5 6)
('(1 2 3) + atom '(a) nil '(dotted . list)) → (1 2 3 a dotted . list)
-
(list reverse) - creates new list with the reverse place of elements. Cycled list is not supported.
-
('(1 2 3 . tail) reverse)→ (3 2 1)
-
(list cycledp) – Cycled list check. The execution time is O(n).
-
(nil setq l '(1 2 3))
(l cycledp) → false
(((l rest)rest)setrest l) → (3 1 2 .. 3 1 2 ... )
(l cycledp) → (1 2 3 .. 1 2 3 ... )
-
(list remove elt) - deletes from the list elements satisfying to equality (elt = listi), returns the new list without the given elements
-
('(1 2 3) remove 2) → (1 3)
-
(list substitute old new) - replacement of elements old on new (at comparison the method = is used). Returns the new list.
-
('(a x x a) substitute 'x 'b) → (a b b a)
(cons copy) - one link of list copy.
(list copy-list) - returns the new list. Cells of top level are copied only. A copying of the looped lists is not supported. The execution time is O(n).
(list copy-list-protected) - returns the new list. Cells of top level are copied only. A copying of the looped lists is supported. The execution time is O(n²).
(list copy-tree) - returns a new tree. Cells, both first and rest are copied all.
-
(list subseq [indexfirst [indexend]]) - with no arguments returns copy of the list. With one argument, returns a new list of elements from index indexfirst. Indexes are integers, numbering starts from zero. The second index represents the limit. List item at the given index in the result will not be.
-
('(0 1 2 3) subseq) → (0 1 2 3)
('(0 1 2 3) subseq 1) → (1 2 3)
('(0 1 2 3) subseq 1 2) → (1)
-
(list without [iteratorlimit]) - without an argument returns a copy of the list. Returns a new list of elements starting from the beginning without the items presented by argument. Iterator is the ultimate cell list.
-
(nil setq l '(a b c d))
('i set (l rest)) → (b c d)
(l without i) → (a)
('j set (i rest)) → (c d)
(l without j) → (a b)
-
(cons setfirst newfirst) - replaces a head part of the list cons with object newfirst
-
(list setrest newrest) - replaces a tail part of the list list with object newrest. If list an element of your program it is necessary to know, that these procedures change the program and it is hard to present as she then looks. That you did not have mistakes use in the program not (nil let ((l '(nil progn ) … but (nil let ((l (list nil 'progn)…
-
(cons cons first rest)=(cons setfirst first),(cons setrest rest)
(cons cons another-cons)=(cons setf another-cons)
-
(consthis setf consarg)=(consthis setfirst (consarg first)),(consthis setrest (consarg rest)) - replaces parts of a cell on new, given consarg. Use of the given operation is fraught with mistakes of programming.
-
(consthis swap consarg) - change the cell references of list. Use of the given operation is fraught with mistakes of programming.
-
(list += [{list}]) - all lists unites, destroys their structure, except for the last. Returns the united list. The looped lists is not supported.
-
('list1 set '(a b . c))
('list2 set '(c d . e))
('list3 set '(e f . g))
(list1 += list2 list3)
list1 → (a b c d e f . g)
list2 → (c d e f . g)
list3 → (e f . g)
-
(list for* symbol {body}) - by turns local variable symbol gives elements of the list list , forms body are calculated by turns. Last result of the form body comes back.
-
('(1 2 3) for* i (cout writeln "i=" i "..")) →
i=1..
i=2..
i=3..
To stop the cycle inside the body more comfortable using the exceptions.
(nil try (list for* … (nil throw 'break) … ) break last-task)
-
(list for symbol {body}) - to in parallel local variables symbol (for each process the variable symbol) elements of the list are given, at each value forms body are calculated by turns. The looped lists is not supported.
-
('(1 2 x) for i (nil if (nil not (nil numberp i)) (cout writeln i " is not number"))) →
x is not number
nil
To stop the cycle inside the body more comfortable using the exceptions.
(nil try (list for … (nil throw 'break) … ) break last-task)
(list assoc who [testeql]) - returns a pair (obj . obj-value) from a list ((a . 1) (b . 2) … (c . 3)) at which who=obj if this pair there exists, and nil - at absence of value. By search predicates of equality of objects testeql, by default are used: =
-
(list rassoc who [testeql]) - returns a pair (obj . obj-value) from a list ((a . 1) (b . 2) … (c . 3)) at which who=obj-value if this pair there exists, and nil - at absence of value. By search predicates of equality of objects testeql, by default are used: =
-
('((1 . a) (2 . b) (3 . c)) rassoc 'c)→ (3 . c)
(nil acons x y a-list)=(nil cons (nil cons x y) a-list) - adds in the associative list a pair (x . y), the result should be saved: ('a-list set (nil acons x y a-list))
-
(a-list pairlist keys-list data-list) - returns the associative list from the list of keys and the list of data, and also adds old a-list.
-
('((a . 1)) pairlist '(b c) '(2 3)) → ((b . 2) (c . 3) (a . 1))
(list = list … list) - comparisons of the contents of all lists
(list <> l1 … ln) - any list li is not equal list.
-
(list contain item [test]) - whether is item in list, by default test is =. Returns list element or false.
-
('(a b c d) contain 'c) → (c d)
(list vector) - returns a new vector with elements of the list
(nil vector) - returns a new empty vector #()
-
(list map fn [{list}]) - application of function fn to elements of list and elements of [{list}]. Value of a variable fn can be a name or lambda-list. The list of result is returned. Calculations executed in parallel.
-
('(1 2 3 4) map '- '(1 1 1 1)) → '(0 1 2 3)
('(1 2 3 4) map '-) → (-1 -2 -3 -4)
('(1 2.3 x "4") map '(lambda () (nil numberp this))) → (1 2.3 false false)
-
(list mapc fn [{list}]) - application of function fn to elements of list and elements of [{list}]. Value of a variable fn can be a name or lambda-list. Does not return value. It is used for side effect obtaining. Calculations executed in parallel.
(list count [{x}]) - quantity of units of the list satisfying to inquiry (elt = [{x}]).
(list count-if fn [{x}]) - quantity of units of the list satisfying to inquiry (elt fn [{x}]).
-
(list find [{x}]) - the list cell containing a unit satisfying condition (elt = [{x}]) comes back.
-
('(1 2 3) find 2) → (2 3)
('(1 2 3) find 4) → nil
-
(list find-if fn [{x}]) - the list cell containing a unit satisfying condition (elt fn [{x}]) comes back.
-
('(1 2 3) find-if 'evenp) → (2 3)
('(1 2 3) find-if '> 10) → nil
-
(list nsubstitute old new) - substitutes list units satisfying to a condition (elt = old) on new value new.
-
(list nsubstitute-if fn new) - substitutes list units satisfying to a condition (elt fn) on new value new.
-
(tree nsubst [{old new}]) - replaces elements of the tree, satisfying (elt = old) to a new value new. The branches of the tree are processed in parallel, the set of rules in sequence.
-
('(x 2 (y x (4 6 7 . x) x 6) 8 9) nsubst 'x 'www 'y -7 'www '(x)) →
((x) 2 (-7 (x) (4 6 7 x) (x) 6) 8 9)
A balanced binary tree
-
('btree newobject method-less) - new empty tree with an argument: the function "less". Easier to pass a lambda function.
-
('btree newobject '(lambda (x) (this < x)))
(btree btree method-less) - devastates the tree changes the function "less".
-
(btree insert x) - adds an element to tree. Upon detection of the presence of "equal" content - changes it. Returns the number of new elements inserted: 1 or 0. Speed O(log(size)).
-
(btree-target insert btree-source) - adding all of the elements in the tree. Upon detection of the presence of "equal" content - changes it. Returns the number of new elements inserted. Speed O(n*log(size)).
-
(btree erase x) - removes an element from the tree. Returns the number of deleted elements: 1 or 0. Speed O(log(size)).
-
(btree erase btree-node) - removes the element of tree supplied. Returns the number of deleted elements: 1. Speed O(log(size)). If the tree does not contain given element, the effect is not defined.
(btree find content) - search for element. Returns the element a tree or nil. Speed O(log(size)).
(btree method-less) - returns the function of the order of the tree.
(btree clear) - devastates the tree. Speed O(1).
(btree size) - returns the number of elements in the tree. Speed O(1).
(btree empty) - predicate of the empty tree. Speed O(1).
(btree copy) - returns a new tree with identical content. Speed O(size).
(btree-target set btree-source) - sets up a tree, with the replacement of the sort function. Speed O(size).
(btree swap btree) - full exchange of content. Speed O(1).
(btree list) - returns a new list of contents of a tree. Speed O(size).
(btree vector) - returns a new vector elements of a tree. Speed O(size).
(btree begin) - returns the element of a tree with "minimal" content. Speed O(log(size)).
(btree end) - returns the element of a tree with "maximum" content. Speed O(log(size)).
(btree root) - returns an element of the tree with the "average" content. Root of the tree. Speed O(1).
The tree consists of the elements. You can use them to view the contents, the transition to the other nodes.
(btree-node content) - returns the contents of a given node. Speed O(1).
(btree-node next) - returns the next node element that is "more" or nil. Speed O(log(size)).
(btree-node prev) - returns the previous node with an element that is "less than" or nil. Speed O(log(size)).
(btree-node left) - returns the node where all items of subtree less than the element of this node. Can return nil. Speed O(1).
(btree-node right) - returns the node where all items of subtree more than the element of this node. Can return nil. Speed O(1).
(btree-node back) - returns the node that leads to the root of the tree. Can return nil, if this node is the root. Speed O(1).
('btree-node newobject father content left right balance)
(btree-node btree-node father content left right balance)
(btree-node insert content method-less)
(btree-node erase content|btree-node method-less)
(btree-node find content method-less)
(btree-node copy)
(btree-node begin)
(btree-node end)
(btree-node list nprocs)
(btree-node vector vector index)
Vector
(vector elt [i [j … [k]]]) - returns an element of vectori,j,…,k. Elements of vector are numbered from 0 up to size-1.
-
(vector size) - returns length of the vector
-
(#() size)→0
(#(1) size)→1
-
(vector resize size [new]) - corrects vectors length in the size , if it is necessary to add the size, additional elements are initialized new.
-
(vector clear)=(vector resize 0)
-
(vector setelt i j … k new) - establishes new value on indexes i,j,…,k on new. Returns corrected vector.
-
(#(1 #(2 3) 4) setelt 1 1 'new) → #(1 #(2 new) 4)
-
(vector reverse) - creates new vector with the reverse place of elements
-
(#(1 2 3) reverse)→ #(3 2 1)
-
('vector newobject dimlist [init]) - creates a vector of dimension dimlist1×dimlist2×…×dimlistn with elements init.
-
('vector newobject '(2 2) 0) → #(#(0 0) #(0 0))
-
('vector newobject number [init]) - creates unidimensional vector of dimension number with elements init.
-
('vector newobject 2 0) → #(0 0)
-
('vector newobject another-vector)=(another-vector copy)
('vector newobject another-vector integer-index-start)=(another-vector subseq integer-index-start)
('vector newobject another-vector integer-index-start integer-index-end)=(another-vector subseq integer-index-start integer-index-end)
('vector newobject) → #()
-
(vector merge {vector}) - returns association of vectors. All vectors do not collapse
-
(#(1 2) merge #() #(3 4)) → #(1 2 3 4)
-
(vector subseq [a [e]]) - With no arguments returns a copy of the vector. Returns a new vector with the elements in the indices since a-th. Parameter e - specifies the starting index from which the data is not copied.
-
(#(1 2 3 4) subseq 1) → #(2 3 4)
(#(1 2 3 4) subseq 1 2) → #(2)
-
(vector push item) - item it is added in vector, vector returns.
(vector push item index) - item is added in index, returns vector
-
('x set #(1 2))(x push 3)→#(1 2 3)
x=#(1 2 3)
('x set #(1 2 3))(x push 7 1)→#(1 7 2 3)
x=#(1 7 2 3)
-
(vector pop [index]) - extract from vector last element and returns it. If given index, then extract element by this index.
-
('y set #(1))
(y push 2) → #(1 2)
(y pop) → 2
y → #(1)
-
(vector contain item [test]) - whether is item in vector, by default test is =. Returns index of founded element or false.
-
(#(a b c d) contain 'c) → 2
(#(a b c d) contain 'e) → false
-
(vector for* symbol {body}) - by turns local variable symbol gives elements of vector, forms body are calculated by turns. Last result of the form body comes back.
-
(#(1 2 3) for* i (cout writeln "i=" i "..")) →
i=1..
i=2..
i=3..
To stop the cycle inside the body more comfortable using the exceptions.
(nil try (vector for* … (nil throw 'break) … ) break last-task)
-
(vector for symbol {body}) to in parallel local variables symbol (for each process the variable symbol ) elements of vector are given, at each value forms body are calculated by turns.
-
(#(1 2 3) for i (cout write " i+1=" (i + 1)) (i + 1)) →
i+1=3 i+1=2 i+1=4
→ nil
To stop the cycle inside the body more comfortable using the exceptions.
(nil try (vector for … (nil throw 'break) … ) break last-task)
(vector copy) - returns a new vector.
(vector copy-matrix) - returns a new vector. If a unit - a vector it also is copied.
-
(vectorthis setf vectorarg) - full replacement of elements of a vector on new, given vectorarg.
(vector vector dimlist [init])≈(vector setf ('vector newobject dimlist [init]))
(vector vector number [init])≈(vector setf ('vector newobject number [init]))
(vector vector another-vector)=(vector setf another-vector)
-
(vector vector another-vector integer-index-start) - assign elements from starting index.
-
(#() vector #(1 2 3) 1) → #(2 3)
-
(vector vector another-vector integer-index-start integer-size) - assign elements from starting index, with given new size.
-
(#() vector #(1 2 3) 1 2) → #(2)
-
(matrix transpose) - returns a new matrix with the rearranged elements
-
(#(#(1 2) #(3 4)) transpose) → #(#(1 3) #(2 4))
-
(vector + {vector}) - addition of vectors of numbers, matrixes and other objects which has method +.
-
(#(1 2) + #(3 -4)) → #(4 -2)
(#(#(1 2) #(3 4)) + #(#(1 0) #(0 -1))) → #(#(2 2) #(3 3))
-
(vector - {vector}) - subtraction of vectors of numbers, matrixes and other objects which has method -. Returns new vector.
-
(#(1 2) - #(3 -4)) → #(-2 6)
(#(1 2) -) → #(-1 -2)
(#(#(1 2) #(3 4)) - #(#(1 0) #(0 -1))) → #(#(0 2) #(3 5))
-
(vector * number … ) - multiplication of elements of a vector to number , returns a new vector.
(vector * vector) - scalar multiplication.
-
(#(1 -2) * 2 -3) → #(-6 12)
(#(1 2) * #(-2 1)) → 0
The vector of vectors means a matrix. The matrix is set by lines. The first element of a vector is the first line of the matrix, the second - the second, etc. Operations + , - , * are set in mathematical sense.
R=M+N | Ri,j=Mi,j+Ni,j |
R=M-N | Ri,j=Mi,j-Ni,j |
R=m*N | Ri,j=m*Ni,j |
-
(vector remove elt) - deletes from the vector elements satisfying to equality (elt = vectori), returns the new vector without the given elements
-
(#(1 2 3) remove 2) → #(1 3)
-
(vector = v1 … vn) - equality vector=v1=…=vn
-
Elements of a vector should have a method <>.
-
(vector <> obj1 … objn) - any argument obji is not equal vector.
-
Elements of a vector should have a method <>.
-
(vector list) - returns the new list of elements of a vector
-
(#(1 2) list) → (1 2)
-
(vector empty) - predicate of an empty vector
-
(#(1 2) empty) → false
(#() empty) → #()
-
(vector insert integer-place x) - insert x in a integer-place.
-
(#(1 2) insert 1 7) → #(1 7 2)
-
(vector += [{vector}])≈(vector setf (vector + [{vector}]))
-
(vector -= [{vector}])≈(vector setf (vector - [{vector}]))
-
(vector *= [{number}])≈(vector setf (vector * [{number}]))
-
(vector swap integer-index integer-index) - interchanges the position elements of a vector. Returns a vector.
(vector swap vector) - changes the contents of the vectors. The change is carried out very quickly.
-
('v set #(1 2 3)) → #(1 2 3)
('w set #(a b)) → #(a b)
(v swap w) → #(a b)
v → #(a b)
w → #(1 2 3)
-
(vector first)=(vector elt 0)
-
(vector last)=(vector elt ((vector size) - 1))
-
(vector map fn [{vector}]) - application of function fn to elements of vector and elements of [{vector}]. Value of a variable fn can be a name or lambda-list. The vector of result is returned. Calculations executed in parallel.
-
(#(1 2 3 4) map '- #(1 1 1 1)) → #(0 1 2 3)
(#(1 2 3 4) map '-) → #(-1 -2 -3 -4)
(#(1 2.3 x "4") map '(lambda () (nil numberp this))) → #(1 2.3 false false)
-
(vector mapc fn [{vector}]) - application of function fn to elements of vector and elements of [{vector}]. Value of a variable fn can be a name or lambda-list. Does not return value. It is used for side effect obtaining. Calculations executed in parallel.
(vector count [{x}]) - quantity of units of a vector satisfying to inquiry (elt = [{x}]).
(vector count-if fn [{x}]) - quantity of units of a vector satisfying to inquiry (elt fn [{x}]).
-
(vector find [{x}]) - the index of a unit of a vector satisfying to a condition (elt = [{x}]) comes back.
-
(#(1 2 3) find 2) → 1
(#(1 2 3) find 4) → nil
-
(vector find-if fn [{x}]) - the index of a unit of a vector satisfying to a condition (elt fn [{x}]) comes back.
-
(#(1 2 3) find-if 'evenp) → 1
(#(1 2 3) find-if '> 10) → nil
-
(vector nsubstitute old new) - substitutes vector units satisfying to a condition (elt = old) on new value new.
-
(vector nsubstitute-if fn new) - substitutes vector units satisfying to a condition (elt fn) on new value new.
-
(vector sort) - sorting of units of a vector (order method <).
-
(#(1 2 3 4 3 2 1) sort) → #(1 1 2 2 3 3 4)
Byte-vector
-
('byte-vector newobject size [init]) - creates byte-vector of size size with elements init.
-
('byte-vector newobject 2 1) → #[1 1]
('byte-vector newobject 2) → #[0 0]
-
('byte-vector newobject another-byte-vector)=(another-byte-vector copy)
('byte-vector newobject another-byte-vector integer-index-start)=(another-byte-vector subseq integer-index-start)
('byte-vector newobject another-byte-vector integer-index-start integer-index-end)=(another-byte-vector subseq integer-index-start integer-index-end)
('byte-vector newobject) → #[]
(byte-vector byte-vector size [initvalue])=(byte-vector resize size [initvalue])
(byte-vector byte-vector size)=(byte-vector resize size 0)
(byte-vector byte-vector another-byte-vector)=(byte-vector setf another-byte-vector)
-
(byte-vector byte-vector another-byte-vector integer-index-start) - assign elements from starting index.
-
(#[] byte-vector #[1 2 3] 1) → #[2 3]
-
(byte-vector byte-vector another-byte-vector integer-index-start integer-size) - assign elements from starting index, with given new size.
-
(#[] byte-vector #[1 2 3] 1 2) → #[2]
(byte-vector copy) - returns a new copy of the byte-vector.
(byte-vector setf another-byte-vector) - total change the size and the data
-
(byte-vector swap integer-index integer-index) - interchanges the position elements of a vector. Returns a vector.
(byte-vector swap byte-vector) - changes the contents of the vectors. The change is carried out very quickly.
-
('v set #[1 2 3]) → #[1 2 3]
('w set #[127 128]) → #[127 128]
(v swap w) → #[127 128]
v → #[127 128]
w → #[1 2 3]
-
(byte-vector size) - returns length of the vector.
-
(#[] size)→0
(#[1] size)→1
(byte-vector elt i [number-result-place]) - returns an element of byte-vectori. Elements of vector are numbered from 0 up to size-1. In the presence of an argument number-result-place the new number is not created: (byv elt ind num)≈(num setf (byv elt ind)).
-
(byte-vector setelt [i [new]]) - establishes new value on index i on new. Returns corrected byte-vector. If the argument new is not specified - set to 0.
-
(#[1 2 3] setelt 1 127) → #[1 127 3]
-
(byte-vector resize [size [new]]) - corrects vectors length to the size, if it is necessary to add the size, additional elements are initialized by new. By default, new items will have the value 0.
-
(byte-vector push-back [new]) – new value is added to the end of the vector. Returns the tweaked byte-vector. If the argument new is not specified - added 0.
-
(#[1 2 3] push-back 127) → #[1 2 3 127]
-
(byte-vector push-front [new]) – new value is added to the beginning of the vector. Returns the tweaked byte-vector. If the argument new is not specified - added 0.
-
(#[1 2 3] push-front 127) → #[127 1 2 3]
-
(byte-vector pop-back [number-result]) –
extracts the value of the end of the vector and returns the value.
In the presence of argument number-result the new number is not created: (vec pop-back num)≈(num setf (vec pop-back)). With an empty vector returns nil.
-
('v set #[1 2 3]) → #[1 2 3]
(v pop-back) → 3
v → #[1 2]
-
(byte-vector pop-front [number-result]) – extracts the value of the beginning of the vector and returns the value.
In the presence of argument number-result the new number is not created: (vec pop-front num)≈(num setf (vec pop-front)). With an empty vector returns nil.
-
('v set #[1 2 3]) → #[1 2 3]
(v pop-front) → 1
v → #[2 3]
-
(byte-vector clear)=(byte-vector resize 0)
-
(byte-vector empty) - the predicate of the empty vector.
-
(#[1 2] empty) → nil
(#[] empty) → #[]
-
(byte-vector back [number-result]) – value at the end of the vector.
In the presence of argument number-result the new number is not created: (vec back num)≈(num setf (vec back)). With an empty vector returns nil.
-
(#[1 2 3] back) → 3
-
(byte-vector front [number-result]) – value at the beginning of the vector.
In the presence of argument number-result the new number is not created: (vec front num)≈(num setf (vec front)). With an empty vector returns nil.
-
(#[1 2 3] front) → 1
-
(byte-vector vector [vector-result]) – returns the new vector of the number of elements byte-vector. At presence of argument vector-result number set there.
-
(#[1 2 3] vector) → #(1 2 3)
('v set #(7 7 7 7)) → #(7 7 7 7)
(#[1 2 3] vector v) → #(1 2 3)
v → #(1 2 3)
-
(byte-vector string [string-result]) – returns a new string of characters, set with the of numbers elements of byte-vector as a code. With the presence of argument string-result characters are set there.
-
(#[49 50 51] string) → "123"
('s set "abcd") → "abcd"
(#[49 50 51] string s) → "123"
s → "123"
-
(byte-vector list) – returns a list of the numbers byte-vector.
-
(#[] list) → nil
(#[1 2 3] list) → (1 2 3)
-
(byte-vector subseq [a [e]]) - With no arguments returns a copy of the vector. Returns a new vector with the elements in the indices since a-th. Parameter e - specifies the starting index from which the data is not copied.
-
(#[1 2 3 4] subseq 1) → #[2 3 4]
(#[1 2 3 4] subseq 1 2) → #[2]
-
(byte-vector contain item [test]) - whether is item in vector, by default test is =. Returns index of founded element or false.
-
(#[10 11 12 13] contain '12) → 2
(#[10 11 12 13] contain '14) → false
Strings
(str size) - returns length of a string
('string newobject number [char]) - creates a string of the given size. It is filled by letters char, by default blanks.
('string newobject another-string)=(another-string copy)
('string newobject another-string integer-index-start)=(another-string subseq integer-index-start)
('string newobject another-string integer-index-start integer-index-end)=(another-string subseq integer-index-start integer-index-end)
('string newobject) → ""
-
(str1 + {strn|char}) - returns association of strings str… all the strings do not collapse
-
('x set "Year") ("New" + " " x #\!)→ "New Year!"
(str0 = str1 … strn) equality str0=str1=…=strn
-
(str <> st1 … stk) - any string sti is not equal str
-
(nil not (str <> st1 … stk)) - string is equal to one of the arguments.
(str0 < str1 … strn), (str0 > str1 … strn), (str0 <= str1 … strn), (str0 >= str1 … strn) - on a code of characters (alphabet order).
(str min str … str), (str max str … str) - minimal/maximal
-
(obj string) - creates a line which represents line performance of object obj.
Transformation of types is supported: nil, boolean, char, number, symbol, byte-vector.
-
(('(1) rest) string) → "NIL"
('abcd string) → "abcd"
-
(str elt ind) - the character on a place ind.
-
If ind exceeds the size of a string comes back nil.
-
(str setelt ind newchar) - puts other character newchar on a place ind. Returns the corrected string str.
-
If ind exceeds the size of a string the string increases the size with addition of spaces.
-
(string subseq [a [e]]) - With no arguments returns a copy of the string. Returns a new string with the elements on the indices since a-th. Parameter e - specifies the starting index from which the data is not copied.
-
("abcd" subseq 1) → "bcd"
("abcd" subseq 1 2) → "b"
(str lower) returns a new string with letters in the bottom register
(str upper) returns a new string with letters in the top register
(str read) - converts a line in Lisp object (the built - in parser)
-
(string for* symbol {body}) - by turns local variable symbol gives elements string string , forms body are calculated by turns. Last result of the form body comes back.
-
("(1 2 3)" for* i (cout writeln "i=" i "..")) →
i=(..
i=1..
i= ..
i=2..
i= ..
i=3..
i=)..
To stop the cycle inside the body more comfortable using the exceptions.
(nil try (string for* … (nil throw 'break) … ) break last-task)
-
(string for symbol {body}) to in parallel local variables symbol (for each process the variable symbol ) elements string string are given, at each value forms body are calculated by turns.
-
("abc" for i (cout write " number(i)=" (i number)) (i number)) →
number(i)=99 number(i)=98 number(i)=97
→ nil
To stop the cycle inside the body more comfortable using the exceptions.
(nil try (string for … (nil throw 'break) … ) break last-task)
-
(string contain char [test]) - whether is char in string, by default test is =
-
("abcd" contain #\c) → 2
("abcd" contain #\e) → false
-
(string list) - returns the list of characters.
-
("Abcd" list) → (#\A #\b #\c #\d)
-
(string vector) - returns the vector of characters.
-
("Abcd" vector) → #(#\A #\b #\c #\d)
-
(string byte-vector) – returns a byte-vector encoding strings in UTF-8.
-
-
(string remove elt) - deletes from the string elements satisfying to equality (elt = stringi), returns the new string without the given elements
-
("ABBA" remove #\B) → "AA"
(string copy) - returns new string.
-
(string setf string) - physically replaces a string with new value. Use of the given operation is fraught with mistakes of programming.
-
('x set "abc") → "abc"
('y set (nil list x x x)) → ("abc" "abc" "abc")
(x setf (x upper)) → "ABC"
y → ("ABC" "ABC" "ABC")
For avoidance of damage of the working program it is necessary to use function of copying:
(nil let ((x "")) … → (nil let ((x ("" copy))) …
('x set "abc") → (x setf "abc")
(nil setq x "abc") → (x setf "abc")
(string swap string) - physically exchange the values.
(string string number [char])≈(string setf ('string newobject number [char]))
(string string another-string)=(string setf another-string)
(string string another-string integer-index-start)=(another-string subseq integer-index-start)
(string string another-string integer-index-start integer-index-end)=(another-string subseq integer-index-start integer-index-end)
-
(string resize newsize [char]) - resize string to newsize. New characters are defined by char. By default is space.
-
(string += [{char|string}])≈(string setf (string + [{char|string}]))
-
(string nsubstitute index size char|string) - substitutes a site of string since index on index+size-1 on new string or the character.
-
("aha" nsubstitute 1 1 "bb") → "abba"
-
(string nsubstitute char char) - substitutes characters.
-
("aha" nsubstitute #\a #\A) → "AhA"
-
(string nsubstitute-if method char) - substitutes characters operations of a method satisfying to result method on new value char.
-
("10x15" nsubstitute-if '(lambda () (nil not (this digitp))) #\space) → "10 15"
(string count [{x}]) - quantity of units of string satisfying to inquiry (elt = [{x}]).
(string count-if fn [{x}]) - quantity of units of string satisfying to inquiry (elt fn [{x}]).
-
(string find [{x}]) - the index of a unit of string satisfying to a condition (elt = [{x}]) comes back.
-
("abcE" find #\E) → 3
("abcE" find #\e) → nil
-
(string find-if fn [{x}]) - the index of a unit of string satisfying to a condition (elt fn [{x}]) comes back.
-
("abcE" find-if 'upperp) → 3
("abcE" find-if 'digitp) → nil
Characters
(char0 = char1 … charn) equality char0=char1=…=charn
-
(char <> char1 … charn) – any chari is not equal to char
-
(nil not ( char <> char1 … charn) - one of the arguments is equal to the object
(char0 <= [char1 … charn]) char0≤char1≤…≤charn
(char0 < [char1 … charn]) char0<char1<…<charn
(char0 > [char1 … charn]) char0>char1>…>charn
(char0 >= [char1 … charn]) char0≥char1≥…≥charn
(char min char … char), (char max char … char) - minimal / maximal
(number char) - returns a character with a code number UTF-16
(ch number) - returns a code of a character ch UTF-16
(ch byte-vector) - returns byte-vector UTF-8.
(byte-vector char) - returns first character from byte-vector UTF-8
(ch alphap) - the letter in the top or bottom register #\a…#\z #\A…#\Z
(ch upperp) - the letter in the top register #\A…#\Z
(ch lowerp) - the letter in the bottom register #\a…#\z
(ch digitp) - #\0…#\9
(ch spacep) - character-divider
(ch controlp) - managing character (ASCII 0…31 or 127)
(ch printp) - whether it is possible to print out
(ch upper) - equivalent of the letter in the top register
(ch lower) - equivalent of the letter in the bottom register
-
(charthis setf chararg)=(charthis char [chararg]) - changes value of the letter on new, given chararg. Use of the given operation is fraught with mistakes of programming.
-
(charthis swap chararg) - changes the values of the letters. Use of the given operation is fraught with mistakes of programming.
-
(char copy) - returns new character.
-
('char newobject char)
('char newobject) - not defined.
Locks and signals
It is used for exclusive access to the important sites of the program.
('lock newobject) - creates the lock.
(lock progn {body})=(nil progn (lock set) {body} (lock unlock)) - the lock is put and by turns calculates tasks body.
After performing all the tasks locking is released. If the lock has been closed, its opening is expected.
(lock try task-locked task-free) - at successful installation of the lock the task-locked is calculated, differently task-free is calculated.
(lock set) - the lock is put. If the lock has been closed, its opening is expected.
(lock unlock) - the lock opening. If the lock was opened, an exception will be thrown.
('signal newobject) - creates the object a signal.
-
(signal wait [lock]) - the signal waiting. If the lock is given then it is disabled and at signal reception is installed.
-
(lock progn
…
(signal wait lock)
…)
(signal send) - dispatches a signal.
Object-oriented programming
This technics of programming that is created that people could understand very big program physically. And the part of a code which has some functions of dialogue with other part of the program is called as object.
All built-in types have the class, it is possible to find out its name with the help of function (nil type-of obj).
(classname defclass) - minimal yet empty definition. Returns classname.
-
(classname defclass pclass1 … pclassn) - definition of a class with parental classes pclass1… pclassn
-
The overload of methods/static variables occurs from left to right:
Methods from pclassn overload pclassn-1 ,… methods of the given class overload all others.
-
(classname defvar [{varname}]) - adds a fields to a class. Symbols varname - are not calculated. Returns classname.
-
External access up to variables of object no. It is possible only with the help of methods.
(classname defstatic varname startvalue) - adds/change a static field of a class. All arguments are calculated.
-
(classname defmethod methodname (… args …) {methodbody}) - adds a methods to the class. With names : methodname and classname::methodname . Tasks methodbody will be calculated by turns.
-
As against the majority of languages, it is possible to dynamically change methods during evaluation of the program - and all existing objects will change accordingly their "behaviour".
(classname defmacro macroname (… args …) {macrobody}) - adds a macroses to a class. With names : macroname and classname::macroname .
-
To define the closed function for work in object it is possible by means of the static variables defined as the lambda-list.
-
('className defstatic 'functionName '(lambda ( … arguments … ) function-body))
-
To start such function it is necessary to use funcall:
-
(nil funcall this functionName … arguments … )
(classname getmethod methodname) - returns definition of a method. methodname is calculated. If such method is not present, nil.
(classname getclass) - returns definition of a class. If such class is not present, nil.
Using:
(obj) → obj
(obj method arg1 … argn) - call of a method of object obj.
Based on object type.
(obj classname::method arg1 … argn) - static. Calling method of base classname.
-
(classname newobject {args}) - creates new object of the given class.
-
If has been created the method with a classname this constructor with arguments {args} is started.
(object copy) - returns new object.
-
(nil private [object [{tasks}]]) - perform tasks within an object with full access to the links of variables. This function can be called only inside the method of this class.
-
('cl defclass)
('cl defvar v)
('cl defmethod cl (x)
(nil setq v x))
('cl defmethod v () v)
('cl defmethod move (x)
(nil setq v
(nil private x
(nil let ((vold v))
(nil setq v nil)
vold))))
(nil setq a ('cl newobject 1) b ('cl newobject 2))
(a v)→1
(b v)→2
(a move b)
(a v)→2
(b v)→nil
Work with streams of an input/output
There are three standard streams which are used by functions by default. They are stored in variables cin, cout and cerr.
(stream write obj … obj) - prints value of obj in convenient kind for viewing the person. Returns stream.
(stream princ obj … obj) - prints values obj as, suitable for input in the interpreter. Returns stream.
(stream writeln obj … obj) - calls function write and terminates a line. Returns stream.
(stream print obj … obj) - calls function princ and terminates a line. Returns stream.
-
(string open [{mode}]) - opens a file with a name string and returns the stream connected with it. The mode of a stream is set by means of symbols mode. By default it is considered on reading and writing.
-
- The mode can be set by symbols:
-
in | on reading |
out | on writing |
in-out | on reading and writing |
trunc | to cut down a file till zero length |
app | (append) addition in the end (but only) |
ate | (at end) opening and search of the end of a file (you can move backwards) |
async | enable signal-driven I/O |
cloexec | enable the close-on-exec flag for the new file descriptor |
direct | File I/O is done directly to/from user-space buffers. |
directory | If string is not a directory, cause the open to fail. |
dsync | Write operations on the file will complete according to the requirements of synchronized I/O data integrity completion, but will only flush metadata updates that are required to allow a subsequent read operation to complete successfully. |
sync | Write operations on the file will complete according to the requirements of synchronized I/O data integrity completion. |
excl | Ensure that this call creates the file: if this flag is specified in conjunction with create, and file already exists, then open will fail. |
largefile | (LFS) Allow files whose sizes cannot be represented in an 32 bit type. |
noatime | Do not update the file last access time (st_atime in the inode) when the file is read. |
noctty | If string refers to a terminal device it will not become the process's controlling terminal even if the process does not have one. |
nofollow | If string is a symbolic link, then the open fails. |
nonblock or ndelay | When possible, the file is opened in nonblocking mode. |
path | Obtain a file descriptor that can be used for two purposes: to indicate a location in the filesystem tree and to perform operations that act purely at the file descriptor level. |
create | if the file does not exist, it will be created ( out and trunc ) |
tmpfile | Create an unnamed temporary file. The string specifies a directory; an unnamed inode will be created in that directory's filesystem. |
- For the create and tmpfile modes, you must set the permissions to access the file for future.
-
rwxusr | 00700 user (file owner) has read, write, and execute permission |
rusr | 00400 user has read permission |
wusr | 00200 user has write permission |
xusr | 00100 user has execute permission |
rwxgrp | 00070 group has read, write, and execute permission |
rgrp | 00040 group has read permission |
wgrp | 00020 group has write permission |
xgrp | 00010 group has execute permission |
rwxoth | 00007 others have read, write, and execute permission |
roth | 00004 others have read permission |
woth | 00002 others have write permission |
xoth | 00001 others have execute permission |
suid | 04000 set-user-ID bit ( Set owner User ID up on execution ) |
sgid | 02000 set-group-ID bit ( Set owner Group user ID up on execution ) (In directory all new files takes group of this directory) |
svtx | 01000 sticky bit (in gived directory to remove file may only owner of this file) |
(string create)=(string open 'out 'trunc) - creates a new empty file with a name string and returns the stream connected with it for writing.
(stream close) - closes a stream. Returns nil at success or one of the symbols badf, intr, io at error.
(stream read) - reads from a stream string representation of object and returns Lisp2D object.
-
(stream read-line [string]) - reads a line of characters from a stream and returns a string without a character of a line termination. If the string is given result is placed there. When picked end of file returns nil.
-
(cin read-line) - from the console
(stream read-char [char]) - reads 1 character from a stream. If it will be given char result it is placed there. Returns the character or nil.
(stream read-byte [number]) - reads 1 byte from a stream and returns an integer. If the number is given result is placed there. Returns the number 0..255 or nil.
(stream listen) - returns true when in a stream not found out the sign of the end of a file, else - false
(stream openp) - returns stream when stream is open, else - false.
(stream terpri) - writes a symbol of the end of a line to a stream
(stream write-byte integer|byte-vector) - writes to a stream 1 byte or byte-vector bytes. Returns number of bytes writened or error symbol (one of the again, badf, destaddrreq, dquot, fault,
fbig, intr, inval, io, nospc, perm, pipe, wouldblock).
(stream tell) - returns an integer - a position of reading/writing of a stream
-
(stream seek number [mode]) - establishes a position of writing/reading in a stream. Returns number - position in a stream.
-
-
The first byte has a position 0 , last size-1
The symbol mode sets a mode of readout of a position:
-
beg | from the beginning of a current file |
cur | from a current position plus number |
end | from the end of a current file plus number |
hole | The first available empty place in the file after the specified position or the end |
data | The first place filled by data in the file |
(stream flush) - clears the buffer of a stream. Returns nil on success or symbol io | rofs | inval | badf | fail | nobuf.
(string|stream truncate size) - changes the size of a file given by the name string or stream. Returns error symbol (access, fault, fbig, intr, inval, io, isdir, loop, nametoolong, noent, notdir, perm, rofs, txtbsy, badf) or nil, if alright.
-
(string|stream chdir) - makes current the given catalogue. Returns nil or error symbol access, badf, fault, io, loop, nametoolong, noent, nomem, notdir.
- (string-filename|stream change-owner-group [number-UID|nil [number-GID|nil]]) - install the identifier of the owner and group of a file (if let link then to a link address). At given nil owner/group is not modified. (Simple user has not access to modify this). Returns nil or symbol of error (access, fault, loop,
nametoolong, noent, nomem, notdir, perm, rofs, badf, io).
-
("." change-owner-group 1000 100) → nil
("." change-owner-group nil 101) → perm
("." change-owner-group 1001) → perm
- (string-filename lchange-owner-group [number-UID|nil [number-GID|nil]]) - install the identifier of the owner and group of the link itself (if it is an usual file then to a file). At given nil owner/group is not modified. (Simple user has not access to modify this). Returns nil or symbol of error (access, fault, loop,
nametoolong, noent, nomem, notdir, perm, rofs, badf, io).
Stat - file status
File structure. It is taken using a path or stream.
-
(stat stat [stat|string-path|stream]) - constructor
('stat newobject) - new empty structure
('stat newobject stat) - new copy
('stat newobject string-path)=(string-path stat)
('stat newobject stream)=(stream stat)
-
(string-path stat [stat-place]) - returns file status along path string-path. If the path is a link, then the status of the file to which the link points. If the argument is given stat-place, then the result is written in this place. On error, the symbol is returned ( access , badf , fault , loop , nametoolong , noent , nomem , notdir , overflow ) .
(string-path lstat [stat-place]) - returns file status along path string-path. If the path is a link, then the status of the link itself is returned. If the argument is given stat-place, then the result is written in this place. On error, the symbol is returned ( access , badf , fault , loop , nametoolong , noent , nomem , notdir , overflow ) .
(stream stat [stat-place]) - returns the status of stream. If the argument is given stat-place, then the result is written in this place. On error, the symbol is returned ( access , badf , fault , loop , nametoolong , noent , nomem , notdir , overflow ) .
(stat setf [statx]) - in struct stat the data statx is copied. The object itself returned.
(stat swap [statx]) - data exchange structures stat and statx. The object itself returned.
- (stat device) - list of numbers ( major minor ).
- (("lisp2d" stat) device) → (9 0)
- (sttat rdevice) - list of numbers ( major minor ). The identifier of the device (for special file).
-
('s set ("lisp2d" stat))
(s device) → (9 0)
(s rdevice) → (0 0)
('s set ("/dev/tty" stat))
(s device) → (0 6)
(s rdevice) → (5 0)
- (stat inode) - number of index unit.
- (("lisp2d" stat) inode) → 1714521
- (stat permissions) - permission list, may contain symbols svtx , sgid ,
suid , xoth , woth , roth , xgrp , wgrp , rgrp , xusr ,
wusr , rusr . see rights
- (("." stat) permissions) → (xoth roth xgrp rgrp xusr wusr rusr)
- (stat file-type) - symbol, file type. ( socket , link , regular , block , directory , character , fifo , ? ) .
- (("." stat) file-type) → directory
- (stat links) - number of hard links.
- (("lisp2d" stat) links) → 1
- (stat uid) - number, the identifier of the user of a file.
- (("lisp2d" stat) uid) → 1001
- (stat gid) - number, the identifier of group of a file.
- (("lisp2d" stat) gid) → 100
- (stat totalsize) - the size of a file in bytes.
- (("lisp2d" stat) totalsize) → 1198880
-
(stat blocksize) - the optimal size of the block for operations of input-output.
-
(("." stat) blocksize) → 4096
-
(stat blocks) - the general number of blocks with 512 bytes.
-
(("." stat) blocks) → 8
-
(stat access-time) - time of last access. Returns object of class time.
-
(stat modify-time) - time of last modification. Returns object of class time.
-
(stat change-time) - time of last status change. (i.e. : owner, group, links count, mode, etc.) Returns object of class time.
Windows
-
('window newobject width height) - creates a window with width and height. Returns object for job with a window.
-
(window window [width height]) – constructor.
(win close) - closes a window
Coordinates are defined from left to right and from top to down.
(nil mapwindow width height) - creates a window without monitoring in display.
(window-mapped open) - show the window on display. Returns window or false.
(window openp) - is it open.
(window hide) - close the window. The image is keeped.
The drawing of the image with the closed window is much faster.
(win rename string) - gives a title to window.
(win move x y) transfers a window to coordinate (x y)
(win resize w h) changes the sizes of a window and a matrix of colors
(win map-resize width height) – changes the size of color matrix.
-
(nil display-size) returns the sizes of the display in pixels as the list of numbers (width height)
-
(nil display-size) → (1280 1024)
-
(nil display-sizemm) returns the sizes of the display in millimetres as the list of numbers (width height)
-
(nil display-sizemm) → (342 271)
(nil screen-count) – returns an integer.
(win width) - width of a window in pixels
(win height) - height of a window in pixels
(window pointer-coord) - returns local coordinates of the pointer as the list (x y).
-
(window wait-event) - waits for an event in a window and returns it.
-
Events are provided by list where the first element is provided as the symbol. With each event may be given auxiliary information. Supported such events:
-
(button-press button x y) - pressing the mouse button. button - button number: 1..7. x,y - coordinate of the mouse pointer at the time of the event.
-
(button-release button x y) - releasing the mouse button. button - button number: 1..7. x,y - coordinate of the mouse pointer at the time of the event.
-
(key-press char|number) - pressing the keyboard. Returns char, if the button provides a letter in UTF-8, otherwise number as the control key code. Key codes are given the values of global variables: xk-enter, xk-home, xk-end, xk-left, xk-right, xk-up, xk-down, xk-page-up, xk-page-down, xk-insert, xk-delete, xk-caps-lock, xk-shift-l, xk-shift-r, xk-control-l, xk-control-r, xk-alt-l, xk-alt-r, xk-f1, xk-f2, xk-f3, xk-f4, xk-f5, xk-f6, xk-f7, xk-f8, xk-f9, xk-f10, xk-f11, xk-f12, xk-backspace, xk-undo, xk-num-lock, xk-kp-multiply, xk-space, xk-numbersign, xk-question
-
(key-release) - releasing the button of keyboard.
-
(window-close) - keypress close window.
-
(configure-notify width height) - changing the window size.
(window check-event) - attempts to load the events in the window, in the absence of event returns nil.
(window make-event ev) - adds an event to window. Returns the event.
(window pending) - returns the number of window events already accumulated.
(win getpixel x y) returns color of a point as (r g b), at coordinates outside a window returns nil
(win draw-pixel col|(colr colg colb) x y x y … x y) - Draws points on the screen the set color [0…1] If color puts number will be a grey shade, differently ([0…1] [0…1] [0…1])=(red green blue)
At coordinate x the whole part undertakes only, are from 0 up to width-1
At coordinate y the whole part undertakes only, are from 0 up to height-1
Such sense of coordinates only for working with pixels.
At functions of a portrayal not for job with pixels of coordinate in a window it is numbers from a floating point from 0 up to width (height) . Start of functions of a portrayal at coordinates behind these limits does not result in a mistake and is ignored.
(win draw-square col|(colr colg colb) x y w h … x y w h) Draws the painted over rectangulars the set color [0…1] If color puts number will be a grey shade, otherwise ([0…1] [0…1] [0…1])=(red green blue)
By initial corner it is designated x y , width and height w h.
Coordinates for a portrayal in a window are set by numbers from a floating point from 0 up to width (height) . To paint over all window in one color it is possible to make it so:
(win draw-square col 0 0 w h).
To draw lines on edge of a window it is possible to use:
(win draw-square col 0 0 1 h 0 0 h 1 0 h w -1 w 0 -1 h)
To draw one point:
(win draw-square col x y 1 1)
If coordinates not the whole function draw-square smooths the image. Therefore it is possible to trace many small rectangulars and it gives effect to a half-transparency.
(nil let ((size 200) halfsize shift w (map 10) size/map)
(nil setq
halfsize (size / 2)
shift (size / 20)
size/map (size / map)
w ('window newobject (size + 1) (size + 1)))
((map + 1) for i
(w draw-square '(0 1 1)
0 (i * size/map) (size + 1) 1
(i * size/map) 0 1 (size + 1)))
(halfsize for i
(w draw-square '(1 0 0)
(i - shift) (i + shift) halfsize (i / halfsize)))
(halfsize for i
(w draw-square '(0 0 1)
(i + shift) (i - shift) (i / halfsize) halfsize))
(cout write "press a key...")
(nil let (en)
(nil do-while
(nil setq en ((w wait-event) first))
(nil not (nil or (en = 'key-press) (en = 'button-press)))))
(w close))
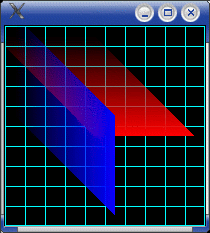
(win draw-line col|(colr colg colb) radius x y x y … x y) - draws lines on the screen the set color [0…1] If color puts number will be a grey shade, differently ([0…1] [0…1] [0…1])=(red green blue). radius - sets thickness of a line, more precisely radius.
Space of names
It is intended for easing an overload of names of functions and constants.
It is recommended to define functions and variables in personal space as if to use other programs and they will redefine function with the same name - that access up to it is impossible.
('name namespace {body}) - connects all constants and functions determined in the given space of names and the body body is carried out by turns.
To define these constants very simply:
('version1 namespace
('conste letset 1000)
(nil defmethod m (x)
(cout write "x=" x)))
('version1 namespace
(nil m conste))
From the outside this space it is impossible to use them:
conste → error
(nil m 1) → error
Almost all functions of language are determined in space of names std and it is possible to cause these functions with the overloaded definition by other programs so:
('another namespace
('number defmethod ! () (cout write "another"))
…
('std namespace
(3 !) → 6
…)
…)
Date and time
-
(nil daylightp) - time of time zone should be corrected with use summertime
-
(nil daylightp) → TRUE
-
(nil setdaylightp flag) - installation of a flag of that time of time zone should be corrected with use summertime
-
(nil timezone) - displacement of time zone in seconds
-
((nil timezone) / 60 60) → -2
-
(nil settimezone sec) - installation displacement of time zone in seconds
-
(nil tzname) - the strings describing time zone
-
(nil tzname) → ("EET" "EEST")
-
(nil settzname string string) - installation of the strings describing time zone
-
(nil time {form}) - calculates by turns the forms form and returns time of calculations in seconds.
-
(nil time 1) → 0.00088
-
(nil localtime) - returns object of class time.
-
(nil localtime) → Time(2008 4 19 17 51 28.0957 6 109 true)
The class of objects time which have methods is defined.
-
(time year)
(time setyear integer)
-
(time month) → 1…12
(time setmonth integer)
-
(time day) → 1…31
(time setday integer)
-
(time hour) → 0…23
(time sethour integer)
-
(time minutes) → 0…59
(time setminutes integer)
-
(time seconds) → 0…59.999999
(time setseconds number)
-
(time weekday) → 0…6
-
0 = sunday
-
(time yearday) → 0…365
-
(time dstp) - daylight saving time. Returns true|false|nil.
-
(time number) - time in seconds past since January, 1, 1970 on UTC (Greenwich). It is used for synchronization on time.
-
(time string)
-
((nil localtime) string) → "Sun Apr 20 19:43:08 2008"
-
(nil uptime) - time in the seconds, past from the moment of loading system
-
(nil uptime) → 8301
-
('time newobject) → Time(1970 1 1 3 0 0 4 0 false)
('time newobject time)=(time copy)
('time newobject number) - installation of time, proceeding from a zero point: on January, 1st 1970. UTC (Greenwich)
-
(time time time)=(time setf time)
(time time number) - installation of time, proceeding from a zero point: on January, 1st 1970. UTC (Greenwich)
-
(time setf time)
-
(time swap time)
-
(time = [{x}]),
(time <> [{x}]),
(time < [{time}]),
(time > [{time}]),
(time <= [{time}]),
(time >= [{time}]),
(time min [{time}]),
(time max [{time}])
Directory
To work with directories there is a class dir.
-
('dir newobject)=('dir newobject ".")
('dir newobject string-path)
(dir dir string-path)=(dir open string-path)
(dir swap dir)
(dir open string-path) - opening of the directory.
(dir openp) - is it opened or not.
(dir close) - directory closing.
(dir read) - returns a string or nil (if files have ended).
-
The program to print contents of the current directory:
-
(nil let (s (d ('dir newobject)))
(nil do-while
(nil if ('s set (d read))
(cout writeln s)))
(d close))
(dir rewind) - cross to the beginning of the directory.
-
(dir seek integer-loc) - cross to a demanded place.
-
integer-loc - is NOT a serial number of an element of the directory.
-
(dir tell) - to receive a current position in the directory.
-
The position is NOT a serial number of an element of the directory.
(string rmdir) - deletes the empty catalogue. Returns nil or error symbol access, busy, fault, inval, loop, nametoolong, noent, nomem, notdir, notempty, perm, rofs.
- (nil getcwd) - string, name of the current catalogue.
- (nil getcwd) → "/home/alex/lisp2d"
-
(string-name mkdir [{access-mode}]) - creates catalogue with access rights. By default the owner has full access, others only view. access-mode may be symbols rwxusr, rusr, wusr, xusr, rwxgrp, rgrp, wgrp, xgrp, rwxoth, roth, woth, xoth, suid, sgid, svtx. see rights
Socket. Networks.
-
('socket newobject)
-
(socket socket)
-
(socket open
domain
type) - returns nil or
string "PROTONOSUPPORT"|"NFILE"|"MFILE"|"ACCES"|"NOBUFS"|"NOMEM"|"INVAL"|"?".
-
domain = ("UNIX" | "LOCAL" | "INET" | "INET6" | "IPX" | "NETLINK" | "X25" | "AX25" | "ATMPVC" | "APPLETALK" | "PACKET")
type = ("STREAM" | "DGRAM" | "SEQPACKET" | "RAW" | "RDM")
-
(socket descriptor) - returns a positive number with the associated socket or -1 there is no connection.
-
(socket close)
-
domain = "UNIX" :
(socket connect string-path) - returns nil or string ( "BADPATH" | "BADF" | "FAULT" | "NOTSOCK" | "ISCONN" | "CONNREFUSED" | "TIMEDOUT" | "NETUNREACH" | "ADDRINUSE" | "INPROGRESS" | "ALREADY" | "AGAIN" | "AFNOSUPPORT" | "ACCES" | "PERM" | "ADDRNOTAVAIL" | "INVAL" | "INTR" | "PROTOTYPE" | "NOSR" | "?" ).
-
Connecting the client, it is desirable to make a cycle with a pause.
(nil let ((socketname (nil const "My Socket")))
(nil let ((sc ('socket newobject)))
(sc open "UNIX" "STREAM")
(nil while (sc connect socketname)
(1 sleep))
…))
-
domain = another :
(socket connect number-port string-addr) - returns nil or string
( "NOADDRSUCCEEDED" | "BADADDR" | "?" | "ADDRFAMILY" | "AGAIN" | "BADFLAGS" | "FAIL" | "FAMILY" | "MEMORY" |
"NODATA" | "NONAME" | "SERVICE" | "SOCKTYPE" | "SYSTEM" | "??" ).
-
(socket bind number-port|string-path) - returns nil or string
"BADF" | "INVAL" | "ACCES" | "NOTSOCK" | "ROFS" | "FAULT" | "NAMETOOLONG" | "NOENT" | "NOMEM" |
"NOTDIR" | "LOOP" | "?"
-
(socket listen n-connections) - returns nil or string
"ADDRINUSE" | "BADF" | "NOTSOCK" | "OPNOTSUPP" | "?"
-
Enabling UNIX server is defined by sequence of calls: create socket-file, open, bind, listen.
(nil let ((socketname (nil const "My Socket")))
(socketname unlink)
(nil let ((sc ('socket newobject)))
(sc open "UNIX" "STREAM")
(sc bind socketname)
(sc listen socket-max-connections)
…
(sc close))
(socketname unlink))
-
(socket accept) - returns the number of userid on success, or on error string
"AGAIN" | "BADF" | "NOTSOCK" | "OPNOTSUPP" | "INTR" | "CONNABORTED" | "INVAL" | "MFILE" | "NFILE" |
"FAULT" | "NOBUFS" | "NOMEM" | "PROTO" | "PERM" | "NOSR" | "SOCKTNOSUPPORT" | "PROTONOSUPPORT" |
"TIMEDOUT" | "?".
-
With the implementation of server the cycle call function accept it is desirable to make on a separate thread.
(nil fork|parallel
(nil while (nil not flagtoquit)
('s set (socket accept))
… ))
-
((( … socket-read … ) ( … socket-write … ) ( … socket-error … )) socket-select [time-out-seconds]) - check the socket's ability to read from them (and/or they expect information) (and/or an error occurred). Returns a list of sockets lists verificated: (( … socket-read … ) ( … socket-write … ) ( … socket-error … )). If is provided an argument time-out-seconds, the function can return from standby and return the result of nil. On error, may return the string "BADF"|"INTR"|"INVAL"|"NOMEM"|"?".
-
(socket read byte-vector-buf [size [{"OOB"|"PEEK"|"WAITALL"|"TRUNC"|"ERRQUEUE"|"NOSIGNAL"}]] ) - receives a message from a socket size of the buffer size, or size . Returns the number - the number of bytes received or string - error "BADF"|"CONNREFUSED"|"NOTCONN"|"NOTSOCK"|"AGAIN"|"INTR"|"FAULT"|"INVAL"|"?".
-
(socket write string [{"OOB"|"DONTROUTE"|"DONTWAIT"|"NOSIGNAL"|"CONFIRM"}] )
(socket write byte-vector-buf [size [{"OOB"|"DONTROUTE"|"DONTWAIT"|"NOSIGNAL"|"CONFIRM"}]] ) - sends a message to a socket size of the buffer size, or size .
- Returns the number - the number of bytes sent or string - error "BADF"|"NOTSOCK"|"FAULT"|"MSGSIZE"|"AGAIN"|"NOBUFS"|"INTR"|"NOMEM"|"INVAL"|"PIPE"|"?".
-
(nil open-protoent)
-
(nil close-protoent)
-
(nil get-protoent) - returns list(number name alias .. alias) or nil
System functions
-
(string-filename system [{string-argument}]) - gives a task to operational system. Returns task terminate code: 0…255 or nil or list (signal number).
-
("ls" system "--help") - task for console.
("mc" system) - starts the program.
-
(string getenv) - variable of environment
-
("USER" getenv) → "alex"
("USEr" getenv) → NIL
("HOME" getenv) → "/home/alex"
-
(nil environ-list) - list of all variables of environment formed by "name=value"
-
(nil environ-list) → ("XDG_VTNR=7" … "_=./lisp2d")
-
(string setenv string) - establishes a variable of environment.
Returns error symbol or nil.
-
("ABC" setenv "DEF")
("ABC" getenv) → "DEF"
(string unsetenv) - reset of value of the variable environment. Returns error symbol or nil.
(nil geteuid) - number, of the identifier of the user
(nil getegid) - number, of the identifier of group
The user root has number of the identifier 0
(nil getuid) - number, of the real identifier of the user
(nil getgid) - number, of the real identifier of group
The user root has number of the identifier 0
(nil sysname) - string, name of operational system, for example "Linux"
-
(nil release) - string, the version of a kernel of operational system
-
(nil release) → "2.6.8.1-12mdk"
-
(nil version) - string, updating of a kernel of operational system
-
(nil version) → "#1 Fri Oct 1 12:53:41 CEST 2004"
-
(nil machine) - string, the platform on which works system
-
(nil machine) → "i686"
-
(nil nodename) - string, name of a computer
-
(nil nodename) → "localhost"
- (nil totalram) - total amount of operative memory in bytes
- ((nil totalram) / 0x400) → 256376
- (nil freeram) - free volume of operative memory in bytes
- ((nil freeram) / 0x400) → 61812
- (string accessf) - check of access or existence of a file
- ("Makefile" accessf) → "Makefile"
("MakeFile" accessf) → FALSE
- (string accessx) - check of executable of the file
- ("lisp2d" accessx) → "lisp2d"
("lisp2d.cpp" accessx) → FALSE
- (string accessr) - check of access on reading of a file
- ("lisp2d" accessr) → "lisp2d"
("lisp2dd" accessr) → FALSE
- (string accessw) - check of access on writing in a file
- ("lisp2d" accessw) → "lisp2d"
("lisp2dd" accessw) → FALSE
- (string-filename setuid number) - install the identifier of user to the file.
- (string-filename setgid number) - install the identifier of user's group to the file.
- (string-filename setluid number) - install the identifier of the user of the reference, instead of the reference object.
- (string-filename setlgid number) - install the identifier of group of the reference, instead of the reference object.
-
(string-filename settime [time-access [time-modify]]) - install access time and time of change of a file. In the absence of arguments current time is installed. Returns error's symbol.
(string-oldpath link string-newpath) - creation of the hard reference to a file, not being the catalogue. Returns error symbol or nil.
(string-oldpath symlink string-newpath) - creates symbolic link.
(string-path readlink) - returns a string of a contents of the symbolic link. At error returns it's symbol.
(string unlink) - deletes a file/removes membership in the catalogue. Returns nil on success or error symbol.
- (string rename string) - changes a name or a position of the file/catalogue.
Returns error symbol or nil.
- ("readme" rename "doc/readme") → nil
- (string ls) - reading of contents of the catalogue, returns the list of names. At error, returns it's symbol.
- ("." ls) → ("." ".." "tmp" ".desktop" "work.cpp" …)
(".." ls) → ("." ".." "alex")
(nil nprocs) - number, quantity of processors of system
(number seteuid) - establishes the working identifier of the user. Returns success: true or false.
-
(number setuid) - establishes the real identifier of the user. Returns success: true or false. Works only from the rights of the superuser.
-
(1002 setuid) → false
(number setegid) - establishes the working identifier of group. Returns success: true or false.
-
(number setgid) - establishes the real identifier of group. Returns success: true or false. Works only from the rights of the superuser.
-
(1002 setgid) → false
-
(string vfsblocksize) - the optimal size of the block for operations of input-output. At failure comes back nil.
-
("." vfsblocksize) → 4096
-
(string frsize) - the fundamental size of the block. At failure comes back nil.
-
("." frsize) → 4096
-
(string vfsblocks) - the general number of blocks. At failure comes back nil.
-
("." vfsblocks) → 4046090
-
(string bfree) - quantity of free blocks for the superuser. At failure comes back nil.
-
("." bfree) → 3308030
-
(string bavail) - quantity of free blocks for the ordinary user. At failure comes back nil.
-
("." bavail) → 3102499
-
(string files) - total of index units. At failure comes back nil.
-
("." files) → 2056320
-
(string ffree) - quantity of free index units for the superuser. At failure comes back nil.
-
("." ffree) → 2036930
-
(string favail) - quantity of free index units for the ordinary user. At failure comes back nil.
-
("." favail) → 2036930
-
(string fsid) - the identifier of file system. At failure comes back nil.
-
("." fsid) → 1218234540
-
(string rdonly) - flag only for reading: true or false. At failure comes back nil.
-
("." rdonly) → FALSE
-
(string nosuid) - the opportunity setuid/setgid is disconnected: true or false. At failure comes back nil.
-
("." nosuid) → FALSE
-
(string namemax) - the maximal length of a name of a file.
At failure comes back nil.
-
("." namemax) → 255
(string setusrreadp flag) - At error returns it's symbol.
(string setusrwritep flag)
(string setusrexecp flag)
(string setgrpreadp flag)
(string setgrpwritep flag)
(string setgrpexecp flag)
(string setothreadp flag)
(string setothwritep flag)
(string setothexecp flag)
(string setuidp flag) - the right to change of the identifier of the user at execution.
(string setgidp flag) - the right to change of the identifier of group at execution.
(string setvtxp flag) - the limited mode of removal from the catalogue.
(number-uid uname) - user name (login).
(number-uid gid) - the numerical identifier of group by default.
(number-uid home) - string, home directory.
(number-uid shell) - command interpreter by default.
(number-gid gname) - group name.
(number-gid gmem) - group members.
(nil getlogin) - the string, user name.
(nil getpid) - returns the process identifier.
(nil getppid) - returns the identifier of parental process.
(string-path chroot) - changes the root catalogue "/". Works only from the rights of the superuser. Returns error's symbol.
(number nice) - change process priority. The new priority or nil comes back. The smaller priority gives more rights.
(nil sethostent stayopen) - start review of database hosts. stayopen - should be true|obj or false|nil. Returns nil. On error returns true.
-
(nil gethostent) - get the next record from the database host. Returns list("the_official_name_of_host" list("alternative_host_names") inet|inet6|integer length_of_each_address list(addresses=list(integers))) or nil.
-
(nil sethostent true) → nil
(nil gethostent) → ("localhost" nil inet 4 ((127 0 0 1)))
(nil endhostent) → nil
(nil endhostent) - complete overview of the database host. Returns nil. On error returns true.
(nil address-of x) - returns the physical address of the object in the computer's memory.
(number-seconds sleep) - falling asleep to the desired number of seconds. It returns the remaining time for certain events, usually 0.
(number-level iopl) - changes the I/O privilege level of the calling process, as specified by the two least significant bits in level. ( number-level = 0 .. 3 ) Returns error : true - if number is not integer or symbol inval, nosys, perm.
(number-port in-byte|in-byte-pause) - returns one byte recieved from port, or symbol inval, io, nomem, perm.
(number-port in-byte-vector byte-vector|size) - returns new byte-vector of size or data filled granted byte-vector. On error returns symbol inval, io, nomem, perm.
(number-port out-byte|out-byte-pause 0..255) - sends one byte in port, returns nil or symbol inval, io, nomem, perm.
(number-port out-byte-vector byte-vector) - sends vector of bytes in port, returns nil or symbol inval, io, nomem, perm.
-
(stream-fd getwinsize) - from the stream reads the window sizes in characters and returns a list (width height). On error, returns one of the characters: notfd , badf , fault , inval , notty.
-
( ( "/dev/stdout" open 'out ) getwinsize ) → (146 39)
Index
&const
&rest
&whole
#\backspace
#\bell
#\escape
#\feed
#\newline
#\null
#\return
#\space
#\tab
#\vtab
!
+ list
+ number
+ string
+ symbol
+ vector
+= cons
+= number
+= string
+= vector
- number
- symbol
- vector
-= number
-= vector
-> imply
* number
* vector
*= number
*= vector
/
/=
= char
= cons
= false
= nil
= number
= string
= symbol
= time
= true
= vector
<> char
<> cons
<> false
<> nil
<> number
<> string
<> symbol
<> time
<> true
<> vector
< char
< number
< string
< symbol
< time
<= char
<= number
<= string
<= symbol
<= time
> char
> number
> string
> symbol
> time
>= char
>= number
>= string
>= symbol
>= time
%
^ expt
∧ and
⊼ nand
∨ or
⊽ nor
⊻ xor
'
`
,
,@
¬ not
∀ for number
λ lambda
√ sqrt
∛ cbrt
abs
accept
accessf
accessr
access-time
accessw
accessx
acons
address-of
alphap
and
apply
arccos
arcosh
arcsin
arctan
arg
arg number
arsinh
artanh
assoc
atom
back btree-node
back byte-vector
backquotep
bavail
begin btree
begin btree-node
bfree
bifp
bind
blocks
blocksize
booleanp
boundp
btree
btreep
btree-node
btree-nodep
button-press
button-release
byte-vector byte-vector
byte-vector char
byte-vector string
byte-vectorp
cbrt
ceil
cerr
change-owner-group
change-time
char byte-vector
char char
char number
charp
chdir
check-event
chroot
cin
clear btree
clear byte-vector
clear vector
close dir
close socket
close stream
close window
close-protoent
combin
combina
cond
configure-notify
conj
connect
cons nil
cons cons
consp
const nil
const symbol
constp nil
constp symbol
contain byte-vector
contain list
contain string
contain vector
content
controlp
copy btree
copy btree-node
copy byte-vector
copy char
copy cons
copy number
copy object
copy string
copy time
copy vector
copy-list
copy-list-protected
copy-matrix
copy-tree
cos
cosh
count list
count string
count vector
count-if list
count-if string
count-if vector
cout
create
cot
coth
cycledp
day
daylightp
defclass
defmacro
defmethod
defstatic
defvar
degrees
denom
descriptor
device
digitp
dir
display-size
display-sizemm
do-while
draw-line
draw-pixel
draw-square
dstp
e
elt byte-vector
elt list
elt string
elt vector
empty btree
empty byte-vector
empty vector
end btree
end btree-node
endhostent
environ-list
environment
environment-external
envp
eq
erase btree
erase btree-node
erf
eval
evenp
exception
exception-history
exp
false
favail
ffree
files
file-type
find btree
find btree-node
find list
find string
find vector
find-if list
find-if string
find-if vector
finitep
first cons
first vector
floor
flush
for list
for number
for string
for vector
for* list
for* number
for* string
for* vector
fork
freeram
front
frsize
fsid
funcall
gcd
gensym
get
get-protoent
getclass
getcwd
getdebug
getegid
getenv
geteuid
getgid
gethostent
getlogin
getmethod
getpid
getpixel
getppid
getuid
getwinsize
gid number
gid stat
gmem
gname
height
hide
home
hour
if
imag
imply
in-byte
in-byte-pause
in-byte-vector
infp
inode
insert btree
insert btree-node
insert vector
integerp
inverf
iopl
key-press
key-release
lambda
last cons
last vector
lchange-owner-group
lcm
left
let
letset
link
links
list btree
list btree-node
list byte-vector
list nil
list string
list vector
list*
listen socket
listen stream
listp
ln
load
localtime
lockp
log nil
log number
log-list
lower char
lower string
lowerp
ls
lstat
machine
make-event
man
map cons
map vector
mapc cons
mapc vector
map-resize
mapwindow
max char
max number
max string
max symbol
max time
merge
method-less
min char
min number
min string
min symbol
min time
minutes
mkdir
mod
modify-time
month
move
multinomial
namemax
namespace
nand
nanp
newobject btree
newobject btree-node
newobject byte-vector
newobject char
newobject cons
newobject dir
newobject lock
newobject number
newobject signal
newobject socket
newobject stat
newobject string
newobject symbol
newobject time
newobject vector
newobject window
next
nice
nil
nodename
nor
nosuid
not
nprocs
nsubst
nsubstitute list
nsubstitute string
nsubstitute vector
nsubstitute-if list
nsubstitute-if string
nsubstitute-if vector
num
number char
number number
number time
numberp
objectp
oddp
open dir
open socket
open string
open window
open-protoent
openp dir
openp stream
openp window
or
out-byte
out-byte-pause
out-byte-vector
package
pairlist
parallel
pending
permissions
pi
pointer-coord
polar
pop
pop-back
pop-front
prev
princ
print
printp
private
progn lock
progn nil
properties
push
push-back
push-front
put
quotep
radians
random
random-normal
randomcomplex
rassoc
rdevice
rdonly
read dir
read socket
read stream
read string
read-byte
read-char
read-eval-print
read-line
readlink
real
release
remove list
remove string
remove vector
remprop
rename string
rename window
resize byte-vector
resize string
resize vector
resize window
rest
reverse list
reverse vector
rewind
right
rmdir
root
round
save
screen-count
second
seconds
seek dir
seek stream
send
set btree
set lock
set symbol
setday
setdaylightp
setdebug
setegid
setelt byte-vector
setelt list
setelt string
setelt vector
setenv
seteuid
setf backquote
setf byte-vector
setf char
setf cons
setf number
setf quote
setf stat
setf string
setf time
setf unquote
setf unquote-splicing
setf vector
setfirst
setgid number
setgid string
setgidp
setgrpexecp
setgrpreadp
setgrpwritep
sethostent
sethour
setlgid
setluid
setminutes
setmonth
setothexecp
setothreadp
setothwritep
setq
setrest
setseconds
settime
settimezone
settzname
setuid number
setuid string
setuidp
setusrexecp
setusrreadp
setusrwritep
setvtxp
setyear
shell
signalp
sin
sinh
size btree
size byte-vector
size cons
size string
size vector
sleep
socket
socket-max-connections
socket-select
socketp
sort
spacep
sqrt
stat stat
stat stream
stat string
statp
streamp
string
string byte-vector
string number
string string
string time
stringp
subseq byte-vector
subseq list
subseq string
subseq vector
substitute
swap backquote
swap btree
swap byte-vector
swap char
swap cons
swap dir
swap number
swap quote
swap stat
swap string
swap time
swap unquote
swap unquote-splicing
swap vector
symbol
symbolp
symlink
sysname
system
tan
tanh
tell dir
tell stream
terpri
third
this
throw
time nil
time time
timep
timezone
totalram
totalsize
transpose
true
trunc
truncate
try lock
try nil
type-of
tzname
uid
uname
unconst nil
unconst symbol
unlink
unlock
unquotep
unquote-splicingp
unsetenv
upper char
upper string
upperp
uptime
vector btree
vector btree-node
vector byte-vector
vector cons
vector nil
vector string
vector vector
vectorp
version
vfsblocks
vfsblocksize
wait
wait-event
weekday
when
while
width
window
window-close
windowp
without
write socket
write stream
write-byte
writeln
xk-…
xor
year
yearday